Default Constructor Java Example
In this post, we feature an example of the Default Constructor in Java.
1. Introduction
When the Java compiler compiles the Java source code (.java) into the Java byte code (.class), it will create a public
no-argument constructor for the class if it has no constructor defined. This public
no-argument constructor is called a default constructor. Its implementation body only has one line of code: super();
– to invoke its parent’s no-argument constructor;
In this step, I will create several classes as the following diagram.
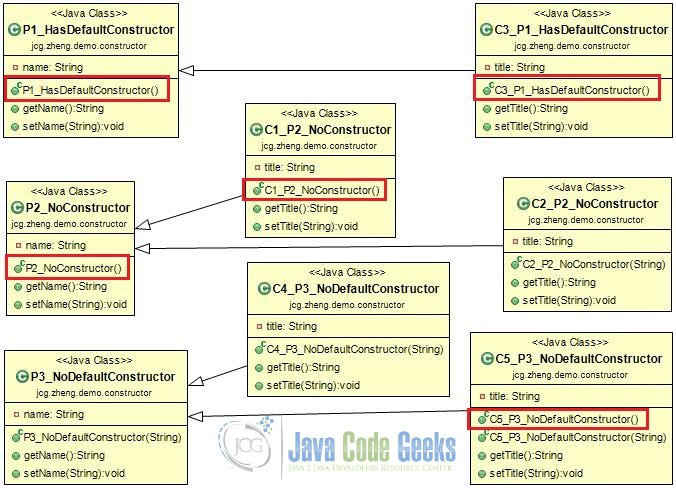
2. Technologies Used
The example code in this article was built and run using:
- Java 11
- Maven 3.3.9
- Eclipse Oxygen
- Junit 5.5.2
3. Maven Project
In this step, I will create eight classes (three parent classes and five child classes):
P1_HasDefaultConstructor
– has a public no-argument constructor.P2_NoConstructor
– doesn’t have any constructor. Java compiler will create a default constructor.P3_NoDefaultConstructor
– has a constructor which takes arguments.C1_P2_NoConstructor
– is a sub-class extending fromP2_NoConstructor
and has no constructor. Java compiler will create a default constructor.C2_P2_NoConstructor
– is a sub-class extending fromP2_NoConstructor
and has a constructor which takes one argument.C3_P1_HasDefaultConstructor
– is a sub-class extending fromP1_HasDefaultConstructor
and has no constructor. Java compiler will create a default constructor.C4_P3_NoDefaultConstructor
– is a sub-class extending fromP3_NoDefaultConstructor
and has a constructor which takes one argument.C5_P3_NoDefaultConstructor
– is a sub-class extending fromP3_NoDefaultConstructor
and has a no-argument constructor.
3.1 Dependencies
I will include Junit
in the pom.xml
.
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>java-zheng-demo</groupId> <artifactId>default-constructor-demo</artifactId> <version>0.0.1-SNAPSHOT</version> <build> <plugins> <plugin> <artifactId>maven-compiler-plugin</artifactId> <version>3.8.0</version> <configuration> <release>11</release> </configuration> </plugin> </plugins> </build> <dependencies> <dependency> <groupId>org.junit.jupiter</groupId> <artifactId>junit-jupiter-engine</artifactId> <version>5.5.2</version> <scope>test</scope> </dependency> <dependency> <groupId>org.junit.jupiter</groupId> <artifactId>junit-jupiter-api</artifactId> <version>5.5.2</version> <scope>test</scope> </dependency> </dependencies> </project>
3.2 P1_HasDefaultConstructor
In this step, I will create a P1_HasDefaultConstructor
class which has a public
no-argument constructor. This is a good practice as client can continue create instances with the no-argument constructor when a new constructor is added.
P1_HasDefaultConstructor.java
package jcg.zheng.demo.constructor; public class P1_HasDefaultConstructor { private String name; public P1_HasDefaultConstructor() { super(); } public String getName() { return name; } public void setName(String name) { this.name = name; } }
3.3 P2_NoConstructor
In this step, I will create a P2_NoConstructor
class which has no constructor. Java compiler will create a default constructor.
P2_NoConstructor.java
package jcg.zheng.demo.constructor; public class P2_NoConstructor { private String name; public String getName() { return name; } public void setName(String name) { this.name = name; } }
3.4 P3_NoDefaultConstructor
In this step, I will create a P3_NoDefaultConstructor
class which has a constructor with an argument. Client cannot create instances with the no-argument constructor.
P3_NoDefaultConstructor.java
package jcg.zheng.demo.constructor; public class P3_NoDefaultConstructor { private String name; public P3_NoDefaultConstructor(String name) { super(); this.name = name; } public String getName() { return name; } public void setName(String name) { this.name = name; } }
3.5 C1_P2_NoConstructor
In this step, I will create a C1_P2_NoConstructor
class which extends from P2_NoConstructor
and has no constructor. Java compiler will create a default constructor.
C1_P2_NoConstructor.java
package jcg.zheng.demo.constructor; public class C1_P2_NoConstructor extends P2_NoConstructor { private String title; public String getTitle() { return title; } public void setTitle(String title) { this.title = title; } }
3.6 C2_P2_NoConstructor
In this step, I will create a C2_P2_NoConstructor
class which extends from P2_NoConstructor
and has a constructor with an argument. Client cannot initialize an object via the no-argument constructor.
C2_P2_NoConstructor.java
package jcg.zheng.demo.constructor; public class C2_P2_NoConstructor extends P2_NoConstructor { private String title; public C2_P2_NoConstructor(String title) { super(); this.title = title; } public String getTitle() { return title; } public void setTitle(String title) { this.title = title; } }
3.7 C3_P1_HasDefaultConstructor
In this step, I will create a C3_P1_HasDefaultConstructor
class which has no constructor and extends from P1_HasDefaultConstructor
. Java compiler will create a default constructor.
C3_P1_HasDefaultConstructor.java
package jcg.zheng.demo.constructor; public class C3_P1_HasDefaultConstructor extends P1_HasDefaultConstructor { private String title; public String getTitle() { return title; } public void setTitle(String title) { this.title = title; } }
3.8 C4_P3_NoDefaultConstructor
In this step, I will create a C4_P3_NoDefaultConstructor
class which has a constructor with an argument.
C4_P3_NoDefaultConstructor.java
package jcg.zheng.demo.constructor; public class C4_P3_NoDefaultConstructor extends P3_NoDefaultConstructor { private String title; public C4_P3_NoDefaultConstructor(String name) { super(name); } public String getTitle() { return title; } public void setTitle(String title) { this.title = title; } }
3.9 C5_P3_NoDefaultConstructor
In this step, I will create a C5_P3_NoDefaultConstructor
class which includes two constructors: one is a no-argument constructor.
C5_P3_NoDefaultConstructor.java
package jcg.zheng.demo.constructor; public class C5_P3_NoDefaultConstructor extends P3_NoDefaultConstructor { private String title; public C5_P3_NoDefaultConstructor() { super("DefaultName"); } public C5_P3_NoDefaultConstructor(String name) { super(name); } public String getTitle() { return title; } public void setTitle(String title) { this.title = title; } }
4. JUnit Test
In this step, I will create two Junit test classes to show two ways to create an object: one using the new
keyword, the other using reflection.
4.1 CreateObjectViaNewTest
In this step, a CreateObjectViaNewTest
class shows how to create an object instance via the new
keyword.
CreateObjectViaNewTest.java
package jcg.zheng.demo.constructor; import static org.junit.jupiter.api.Assertions.*; import org.junit.jupiter.api.Test; import jcg.zheng.demo.constructor.C1_P2_NoConstructor; import jcg.zheng.demo.constructor.C2_P2_NoConstructor; import jcg.zheng.demo.constructor.C3_P1_HasDefaultConstructor; import jcg.zheng.demo.constructor.C4_P3_NoDefaultConstructor; import jcg.zheng.demo.constructor.C5_P3_NoDefaultConstructor; import jcg.zheng.demo.constructor.P1_HasDefaultConstructor; import jcg.zheng.demo.constructor.P2_NoConstructor; import jcg.zheng.demo.constructor.P3_NoDefaultConstructor; public class CreateObjectViaNewTest { private static final String JAVA_DEVELOPER = "Java Developer"; private static final String MARY = "Mary"; @Test public void test_C1_P2_NoConstructor() { C1_P2_NoConstructor pojo = new C1_P2_NoConstructor(); pojo.setName(MARY); assertEquals(MARY, pojo.getName()); } @Test public void test_C2_P2_NoConstructor() { C2_P2_NoConstructor pojo = new C2_P2_NoConstructor(MARY); pojo.setName(MARY); pojo.setTitle(JAVA_DEVELOPER); assertEquals(MARY, pojo.getName()); } @Test public void test_C3_P1_HasDefaultConstructor() { C3_P1_HasDefaultConstructor pojo = new C3_P1_HasDefaultConstructor(); pojo.setName(MARY); pojo.setTitle(JAVA_DEVELOPER); assertEquals(MARY, pojo.getName()); } @Test public void test_C4_P3_NoDefaultConstructor() { C4_P3_NoDefaultConstructor pojo = new C4_P3_NoDefaultConstructor(MARY); pojo.setTitle(JAVA_DEVELOPER); assertEquals(MARY, pojo.getName()); } @Test public void test_C5_P3_NoDefaultConstructor() { C5_P3_NoDefaultConstructor pojo = new C5_P3_NoDefaultConstructor(); pojo.setTitle(JAVA_DEVELOPER); assertEquals("DefaultName", pojo.getName()); } @Test public void test_P1_HasDefaultConstructor() { P1_HasDefaultConstructor pojo = new P1_HasDefaultConstructor(); pojo.setName(MARY); assertEquals(MARY, pojo.getName()); } @Test public void test_P2_NoConstructor() { P2_NoConstructor pojo = new P2_NoConstructor(); pojo.setName(MARY); assertEquals(MARY, pojo.getName()); } @Test public void test_P3_NoDefaultConstructor() { P3_NoDefaultConstructor pojo = new P3_NoDefaultConstructor(MARY); assertEquals(MARY, pojo.getName()); } }
Execute with mvn test -Dtest=CreateObjectViaNewTest
and capture the output here:
Running jcg.zheng.demo.constructor.CreateObjectViaNewTest Tests run: 8, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.045 sec Results : Tests run: 8, Failures: 0, Errors: 0, Skipped: 0
4.2 CreateObjectViaReflectionTest
In this step, a CreateObjectViaReflectionTest
class shows how to create an object instance via the reflection library.
CreateObjectViareflectionTest.java
package jcg.zheng.demo.constructor; import static org.junit.jupiter.api.Assertions.assertNotNull; import static org.junit.jupiter.api.Assertions.assertNull; import java.lang.reflect.Constructor; import java.lang.reflect.InvocationTargetException; import org.junit.jupiter.api.Test; public class CreateObjectViaReflectionTest { private Object createObjectWithNoArgsViaReflection(String className) { try { Class<?> c = Class.forName(className); Constructor<?> publicConstructors = c.getConstructor(); return publicConstructors.newInstance(); } catch (NoSuchMethodException | SecurityException | InstantiationException | IllegalAccessException | IllegalArgumentException | InvocationTargetException | ClassNotFoundException e) { return null; } } private Object createObjectWithStringArgsViaReflection(String className, String constructorArg) { try { Class<?> c = Class.forName(className); Constructor<?> publicConstructors = c.getConstructor(String.class); return publicConstructors.newInstance(new Object[] { constructorArg }); } catch (NoSuchMethodException | SecurityException | InstantiationException | IllegalAccessException | IllegalArgumentException | InvocationTargetException | ClassNotFoundException e) { return null; } } @Test public void test_C1_P2_NoConstructor() { Object object = createObjectWithNoArgsViaReflection("jcg.zheng.demo.constructor.C1_P2_NoConstructor"); assertNotNull(object); } @Test public void test_C2_P2_NoConstructor() { Object object = createObjectWithNoArgsViaReflection("jcg.zheng.demo.constructor.C2_P2_NoConstructor"); assertNull(object); object = createObjectWithStringArgsViaReflection("jcg.zheng.demo.constructor.C2_P2_NoConstructor", "test"); assertNotNull(object); } @Test public void test_C3_P1_HasDefaultConstructor() { Object object = createObjectWithNoArgsViaReflection("jcg.zheng.demo.constructor.C3_P1_HasDefaultConstructor"); assertNotNull(object); } @Test public void test_C4_P3_NoDefaultConstructor() { Object object = createObjectWithNoArgsViaReflection("jcg.zheng.demo.constructor.C4_P3_NoDefaultConstructor"); assertNull(object); object = createObjectWithStringArgsViaReflection("jcg.zheng.demo.constructor.C4_P3_NoDefaultConstructor", "test"); assertNotNull(object); } @Test public void test_S5_Z_NoDefaultConstructor() { Object object = createObjectWithNoArgsViaReflection("jcg.zheng.demo.constructor.C5_P3_NoDefaultConstructor"); assertNotNull(object); } @Test public void test_P1_HasDefaultConstructor() { Object object = createObjectWithNoArgsViaReflection("jcg.zheng.demo.constructor.P1_HasDefaultConstructor"); assertNotNull(object); } @Test public void test_P2_NoConstructor() { Object object = createObjectWithNoArgsViaReflection("jcg.zheng.demo.constructor.P2_NoConstructor"); assertNotNull(object); } @Test public void test_P3_NoDefaultConstructor() { Object object = createObjectWithNoArgsViaReflection("jcg.zheng.demo.constructor.P3_NoDefaultConstructor"); assertNull(object); object = createObjectWithStringArgsViaReflection("jcg.zheng.demo.constructor.P3_NoDefaultConstructor", "test"); assertNotNull(object); } }
Execute mvn test -Dtest=CreateObjectViaReflectionTest
and capture the output here:
Running jcg.zheng.demo.constructor.CreateObjectViaReflectionTest Tests run: 8, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.036 sec Results : Tests run: 8, Failures: 0, Errors: 0, Skipped: 0
5. Default Constructor in Java – Summary
In this example, I demonstrated that Java compiler creates a default constructor when the class does not define any constructor. I also demonstrated how to use Java reflection library to initialize an object. As you seen, it’s easier to create object via reflection when it has a no-argument constructor. Common frameworks, such as JPA and JAXB use Java reflection to create objects. So, it’s the best practice to define the no-argument constructor when using these frameworks.
6. Download the Source Code
You can download the full source code of this example here: Default Constructor Java Example