How to Create a Java Gui with Swing
In this post, we feature a comprehensive tutorial on how to create a Java Gui with Swing. There are two sets of Java APIs for graphics programming: AWT and Swing.
You can also check this tutorial in the following video:
1. Java Gui tutorial with Swing – Introduction
There are two sets of Java APIs for graphics programming: AWT (Abstract Windowing Toolkit) and Swing.
1. AWT
API was introduced in JDK 1.0. Most of the AWT components have become obsolete and should be replaced by newer Swing components.
2. Swing API, a much more comprehensive set of graphics libraries that enhances the AWT, was introduced as part of Java Foundation Classes (JFC) after the release of JDK 1.1. JFC consists of Swing, Java2D, Accessibility, Internationalization, and Pluggable Look-and-Feel Support APIs. JFC was an add-on to JDK 1.1 but has been integrated into core Java since JDK 1.2.
Other than AWT/Swing Graphics APIs provided in JDK, others have also provided Graphics APIs that work with Java, such as Eclipse’s Standard Widget Toolkit (SWT) (used in Eclipse), Google Web Toolkit (GWT) (used in Android), 3D Graphics API such as Java bindings for OpenGL (JOGL) and Java3D.
2. Java GUI Creation
In this tutorial, we will learn how to create a Java GUI with Swing using Eclipse IDE.
2.1 SetUP
Prerequisite:
This example is developed on Eclipse therefore a compatible Eclipse IDE is required to be installed on the system.
We also need WindowBuilder tool to be installed on Eclipse IDE for the easiness of the work.
The following steps are required to install the WindowBuilder tool.
- Go to Eclipse →Help→ Install New Software
- Select your version of eclipse version/download/eclipse.org/release/eclipse version, For example, Mars – http://download.eclipse.org/releases/mars
- Select General purpose tools from the dropdown and click Next.
This will take some time to install the software, so you have to restart eclipse in order to see the changes.
2.2 Programming GUI with AWT
Java Graphics APIs – AWT and Swing – provides a huge set of reusable GUI components, such as button, text field, label, choice, panel and frame for building GUI applications. You can simply reuse these classes rather than re-invent the wheels. I shall start with the AWT classes before moving into Swing to give you a complete picture. I have to stress that AWT component classes are now obsoleted by Swing’s counterparts.
2.2.1 AWT Packages
AWT is huge! It consists of 12 packages (Swing is even bigger, with 18 packages as of JDK 1.8). Fortunately, only 2 packages – java.awt and java.awt.event – are commonly-used.
1. The java.awt package contains the core AWT graphics classes:
- GUI Component classes (such as Button, TextField, and Label)
- GUI Container classes (such as Frame, Panel, Dialog and ScrollPane)
- Layout managers (such as FlowLayout, BorderLayout and GridLayout)
- Custom graphics classes (such as Graphics, Color and Font).
2. The java.awt.event package supports event handling:
- Event classes (such as ActionEvent, MouseEvent, KeyEvent and WindowEvent)
- Event Listener Interfaces (such as ActionListener, MouseListener, KeyListener and WindowListener)
- Event Listener Adapter classes (such as MouseAdapter, KeyAdapter, and WindowAdapter)
AWT provides a platform-independent and device-independent interface to develop graphic programs that runs on all platforms, such as Windows, Mac, and Linux.
2.2.2 Containers and Components
There are two types of GUI elements:
1. Component: Components are elementary GUI entities (such as Button, Label, and TextField.)
2. Container: Containers (such as Frame and Panel) are used to hold components in a specific layout (such as flow or grid). A container can also hold sub-containers.
GUI components are also called controls (Microsoft ActiveX Control), widgets (Eclipse’s Standard Widget Toolkit, Google Web Toolkit), which allow users to interact with (or control) the application through these components (such as button-click and text-entry).
A Frame is the top-level container of an AWT program. A Frame has a title bar (containing an icon, a title, and the minimize/maximize/close buttons), an optional menu bar and the content display area. A Panel is a rectangular area used to group related GUI components in a certain layout. In the above figure, the top-level Frame contains two Panels. There are five components: a Label (providing description), a TextField (for users to enter text), and three Buttons (for user to trigger certain programmed actions).
In a GUI program, a component must be kept in a container. You need to identify a container to hold the components. Every container has a method called add(Component c). A container (say aContainer) can invoke aContainer.add(aComponent) to add aComponent into itself. For example,
GUIProgam.java
1
2
3
4
5
|
//Where the GUI is created: Panel panel = new Panel(); // Panel is a Container Button btn = new Button( "Press" ); // Button is a Component panel.add(btn); // The Panel Container adds a Button Component |
2.2.3 AWT Container Classes
Top-Level Containers: Frame, Dialog and Applet Each GUI program has a top-level container. The commonly-used top-level containers in AWT are Frame, Dialog and Applet:
- A Frame provides the “main window” for the GUI application, which has a title bar (containing an icon, a title, the minimize, maximize/restore-down and close buttons), an optional menu bar, and the content display area. To write a GUI program, we typically start with a subclass extending from java.awt.Frame to inherit the main window as follows:
GUIProgam.java0102030405060708091011121314151617181920212223//Where the GUI is created:
* Launch the application.
*/
public
static
void
main(String[] args) {
EventQueue.invokeLater(new
Runnable() {
public
void
run() {
try
{
GUIProgram window = new
GUIProgram();
window.frame.setVisible(true);
} catch
(Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the application.
*/
public
GUIProgram() {
initialize();
}
- An AWT Dialog is a “pop-up window” used for interacting with the users. A Dialog has a title-bar (containing an icon, a title and a close button) and a content display area, as illustrated.
- An AWT Applet (in package java.applet) is the top-level container for an applet, which is a Java program running inside a browser. Applet will be discussed in the later chapter.
Secondary Containers: Panel and ScrollPane
Secondary containers are placed inside a top-level container or another secondary container. AWT also provide these secondary containers:
- Panel: a rectangular box under a higher-level container, used to layout a set of related GUI components in pattern such as grid or flow.
- ScrollPane: provides automatic horizontal and/or vertical scrolling for a single child component.
2.2.4 AWT Component Classes
AWT
provides many ready-made and reusable GUI components. The frequently-used are: Button
, TextField
, Label
, Checkbox
, CheckboxGroup (radio buttons)
, List, and Choice, as illustrated below. AWT GUI Component:java.awt.Label
A java.awt.Label
provides a text description message. Take note that System.out.println() prints to the system console, not to the graphics screen. You could use a Label to label another component (such as text field) or provide a text description.
Check the JDK API specification for java.awt.Label
.
Below is how a label will look like:
Constructors
GUIProgam.java
1
|
public Label(String strLabel, int alignment); // Construct a Label with the given text String, of the text alignment |
GUIProgam.java
1
|
public Label(String strLabel); // Construct a Label with the given text String |
GUIProgam.java
1
|
public Label(); // Construct an initially empty Label |
The Label class has three constructors:
1. The first constructor constructs a Label object with the given text string in the given alignment. Note that three static constants Label.LEFT, Label.RIGHT, and Label.CENTER are defined in the class for you to specify the alignment (rather than asking you to memorize arbitrary integer values).
2. The second constructor constructs a Label object with the given text string in default of left-aligned.
3. The third constructor constructs a Label object with an initially empty string. You could set the label text via the setText() method later.
GUIProgam.java
1
2
3
|
JLabel lblName = new JLabel( "Name" ); lblName.setBounds( 93 , 67 , 46 , 14 ); frame.getContentPane().add(lblName); |
AWT GUI Component: java.awt.Button
A java.awt.Button
is a GUI component that triggers a certain programmed action upon clicking.
Constructors
GUIProgam.java
1
2
3
4
|
public Button(String buttonLabel); // Construct a Button with the given label public Button(); // Construct a Button with empty label |
The Button class has two constructors. The first constructor creates a Button object with the given label painted over the button. The second constructor creates a Button object with no label.
Here is how the button will look like:
Clicking on a button generates an event. The code for adding a button is described below:
GUIProgam.java
1
2
3
4
5
6
7
8
|
JButton btnSubmit = new JButton( "Submit" ); btnSubmit.addActionListener( new ActionListener() { public void actionPerformed(ActionEvent arg0) { JOptionPane.showMessageDialog( null , "Data Submitted" ); } }); btnSubmit.setBounds( 93 , 121 , 89 , 23 ); frame.getContentPane().add(btnSubmit); |
T GUI Component: java.awt.TextField
A java.awt.TextField is single-line text box for users to enter texts. (There is a multiple-line text box called TextArea.) Hitting the “ENTER” key on a TextField object triggers an action-event.
Constructors
GUIProgam.java
1
2
3
4
5
6
|
public TextField(String strInitialText, int columns); // Construct a TextField instance with the given initial text string with the number of columns. public TextField(String strInitialText); // Construct a TextField instance with the given initial text string. public TextField( int columns); // Construct a TextField instance with the number of columns. |
Below is how a TextField will look like :
3. AWT Event-Handling
Now it’s time to see the AWT Event handling in this Java Gui with Swing tutorial. Java adopts the so-called “Event-Driven” (or “Event-Delegation”) programming model for event-handling, similar to most of the visual programming languages (such as Visual Basic and Delphi).
In event-driven programming, a piece of event-handling codes is executed (or called back by the graphics subsystem) when an event has been fired in response to an user input (such as clicking a mouse button or hitting the ENTER key). This is unlike the procedural model, where codes are executed in a sequential manner.
The AWT’s event-handling classes are kept in package java.awt.event.
Three objects are involved in the event-handling: a source, listener(s) and an event object.
The source object (such as Button and Textfield) interacts with the user. Upon triggered, it creates an event object. This event object will be messaged to all the registered listener object(s), and an appropriate event-handler method of the listener(s) is called-back to provide the response. In other words, triggering a source fires an event to all its listener(s), and invoke an appropriate handler of the listener(s).
The sequence of steps is illustrated above:
- The source object registers its listener(s) for a certain type of event.
Source object fires event event upon triggered. For example, clicking an Button fires an ActionEvent, mouse-click fires MouseEvent, key-type fires KeyEvent, etc.
How the source and listener understand each other? The answer is via an agreed-upon interface. For example, if a source is capable of firing an event called XxxEvent (e.g., MouseEvent) involving various operational modes (e.g., mouse-clicked, mouse-entered, mouse-exited, mouse-pressed, and mouse-released). Firstly, we need to declare an interface called XxxListener (e.g., MouseListener) containing the names of the handler methods. Recall that an interface contains only abstract methods without implementation
Secondly, all the listeners interested in the XxxEvent must implement the XxxListener interface. That is, the listeners must provide their own implementations (i.e., programmed responses) to all the abstract methods declared in the XxxListener interface. In this way, the listenser(s) can response to these events appropriately.
Thirdly, in the source, we need to maintain a list of listener object(s), and define two methods: addXxxListener()
and removeXxxListener()
to add and remove a listener from this list.
Take note that all the listener(s) interested in the XxxEvent must implement the XxxListener interface. That is, they are sub-type of the XxxListener. Hence, they can be upcasted to XxxListener and passed as the argument of the above methods.
In summary, we identify the source, the event-listener interface, and the listener object. The listener must implement the event-listener interface. The source object then registers listener object via the addXxxListener()
method.
- The source is triggered by a user.
- The source create an XxxEvent object, which encapsulates the necessary information about the activation. For example, the (x, y) position of the mouse pointer, the text entered, etc.
- Finally, for each of the listeners in the listener list, the source invokes the appropriate handler on the listener(s), which provides the programmed response.
4. Java Swing class Hierarchy
Java Swing Container classes are used to build screens that have components. The user interface typically needs one container. Java Swing has three types of Container
classes. The container classes will have Window
and JComponent
.The Frame
class is a subclass of Window
. The Panel
class which is a subclass of JComponent
is used to compose the window components. The JFrame
which is a subclass of Frame
class is used for a window which has title and images. The Dialog
class which is a subclass of Window
is similar to a Popup window.
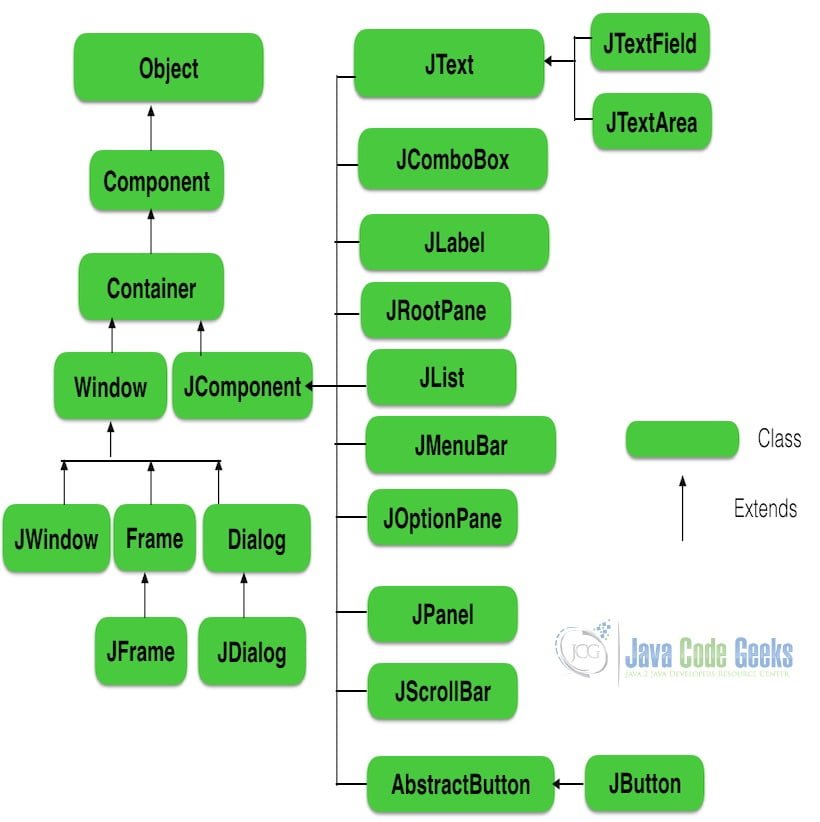
5. Advanced Swing Example
In this section of the Java Gui with Swing article, we will look at a WordFrequency Application tutorial. The application will have a Login screen and WordFrequencyCalculator screen. The login screen code is shown below in the WordFrequencyManager
.
WordFrequencyManager
import javax.swing.*; import java.awt.event.*; /** * @author bhagvan.kommadi * */ public class WordFrequencyManager { /** * @param args */ public static void main(String[] args) { JFrame calculatorFrame=new JFrame("Word Frequency Calculator"); //final JLabel usernameLabel = new JLabel(); //usernameLabel.setBounds(20,150, 200,50); final JPasswordField passwordField = new JPasswordField(); passwordField.setBounds(100,75,100,30); JLabel usernameLabel=new JLabel("Username:"); usernameLabel.setBounds(20,20, 80,30); JLabel passwordLabel=new JLabel("Password:"); passwordLabel.setBounds(20,75, 80,30); final JTextField usernameField = new JTextField(); usernameField.setBounds(100,20, 100,30); JButton loginButton = new JButton("Login"); loginButton.setBounds(100,120, 80,30); calculatorFrame.add(usernameLabel); calculatorFrame.add(usernameField); calculatorFrame.add(passwordLabel); calculatorFrame.add(passwordField); calculatorFrame.add(loginButton); calculatorFrame.setSize(300,300); calculatorFrame.setLayout(null); calculatorFrame.setVisible(true); loginButton.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { WordFrequencyWindow window = new WordFrequencyWindow(); } }); } }
Login screen will have username and password fields. Password will be JPasswordField
type. Username will be JTextField
. The login screen UI will be as in the attached screenshot.
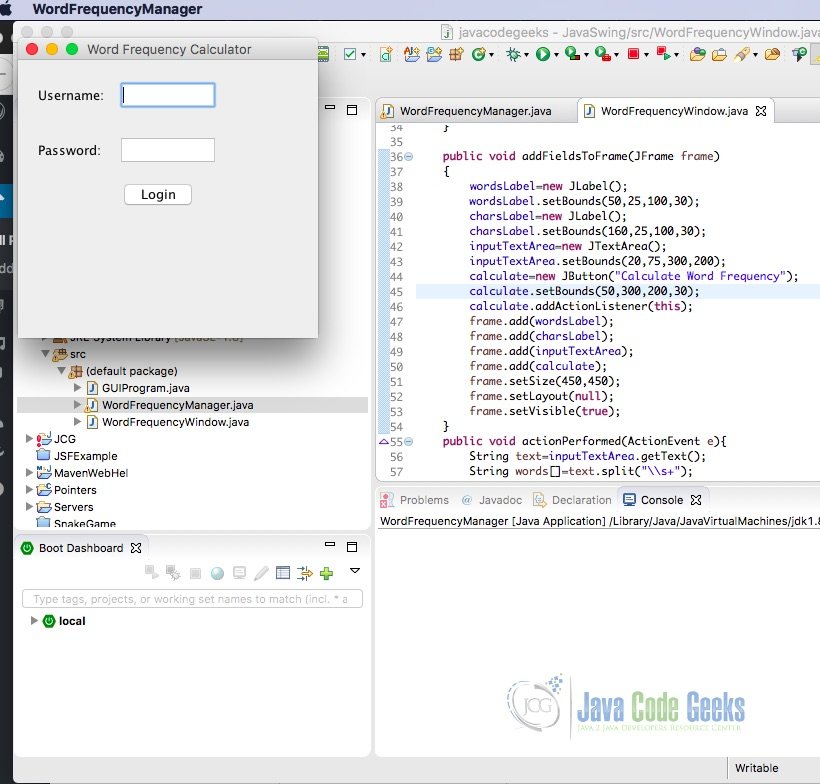
User inputs the username and password for submission. The user will be navigated to the next window. The login screen with filled-in fields will be as shown in the screenshot below.
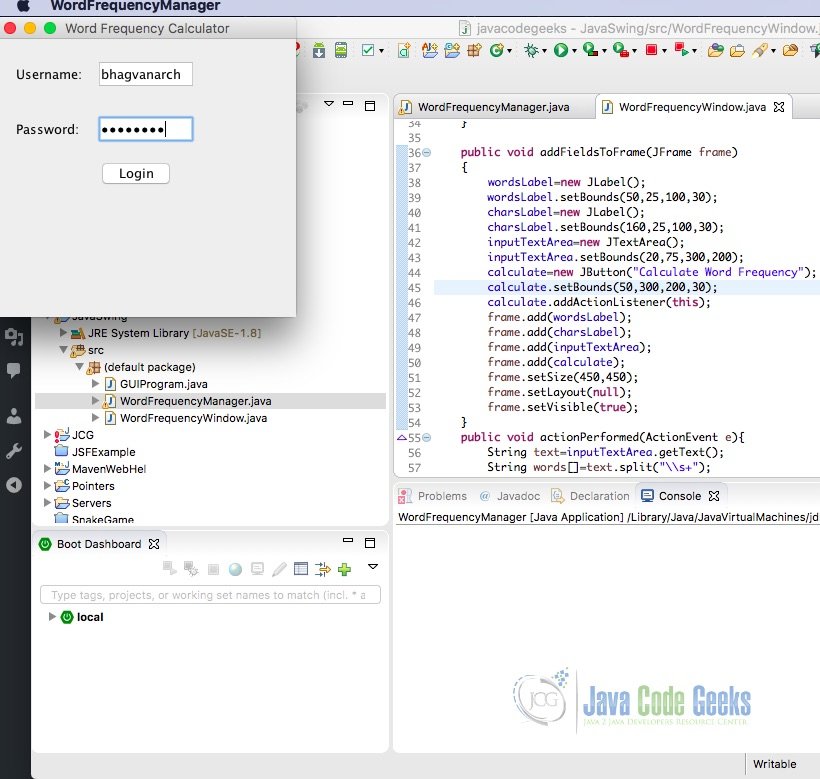
WordFrequencyWindow will have a JTextArea
and Jbutton
fields. WordFrequencyWindow
class source code is as shown below.
WordFrequencyWindow
/** * */ import javax.swing.*; import java.awt.event.*; /** * @author bhagvan.kommadi * */ public class WordFrequencyWindow implements ActionListener{ JLabel wordsLabel,charsLabel; JTextArea inputTextArea; JButton calculate; public WordFrequencyWindow() { JFrame frame= new JFrame(); addCloseListener(frame); addFieldsToFrame(frame); } public void addCloseListener(JFrame frame) { frame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent event) { frame.dispose(); System.exit(0); } }); } public void addFieldsToFrame(JFrame frame) { wordsLabel=new JLabel(); wordsLabel.setBounds(50,25,100,30); charsLabel=new JLabel(); charsLabel.setBounds(160,25,100,30); inputTextArea=new JTextArea(); inputTextArea.setBounds(20,75,300,200); calculate=new JButton("Calculate Word Frequency"); calculate.setBounds(50,300,200,30); calculate.addActionListener(this); frame.add(wordsLabel); frame.add(charsLabel); frame.add(inputTextArea); frame.add(calculate); frame.setSize(450,450); frame.setLayout(null); frame.setVisible(true); } public void actionPerformed(ActionEvent e){ String text=inputTextArea.getText(); String words[]=text.split("\\s+"); wordsLabel.setText("Words: "+words.length); charsLabel.setText("Characters: "+text.length()); } }
The word Frequency window, when launched in eclipse will be as shown in the screenshot below.
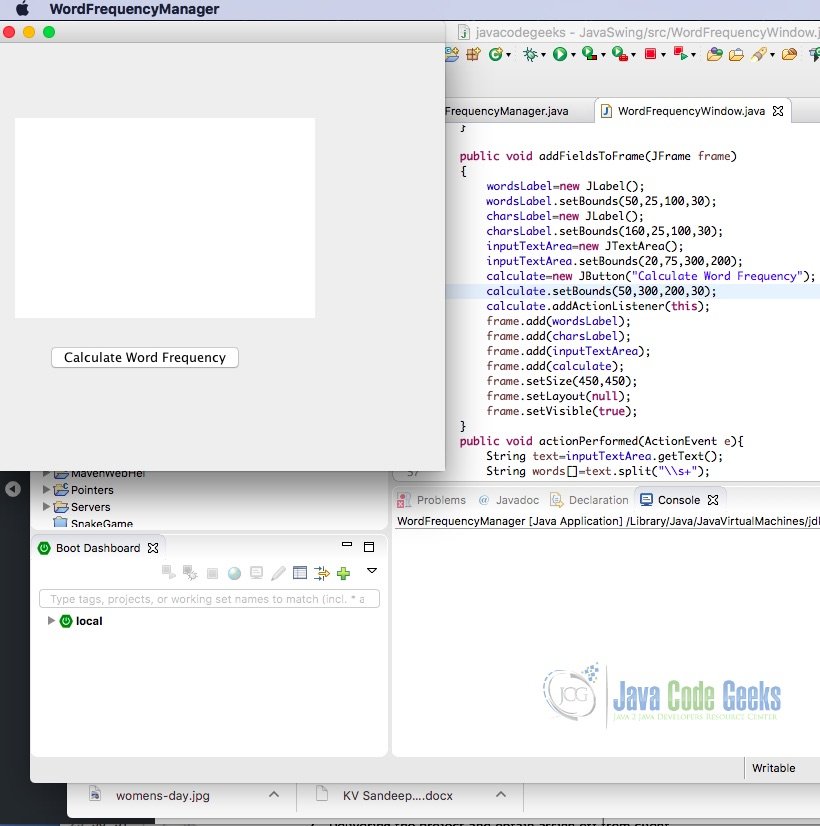
User inputs the text area with a text which has words. The calculator window after input is filled looks as shown in the screenshot below:
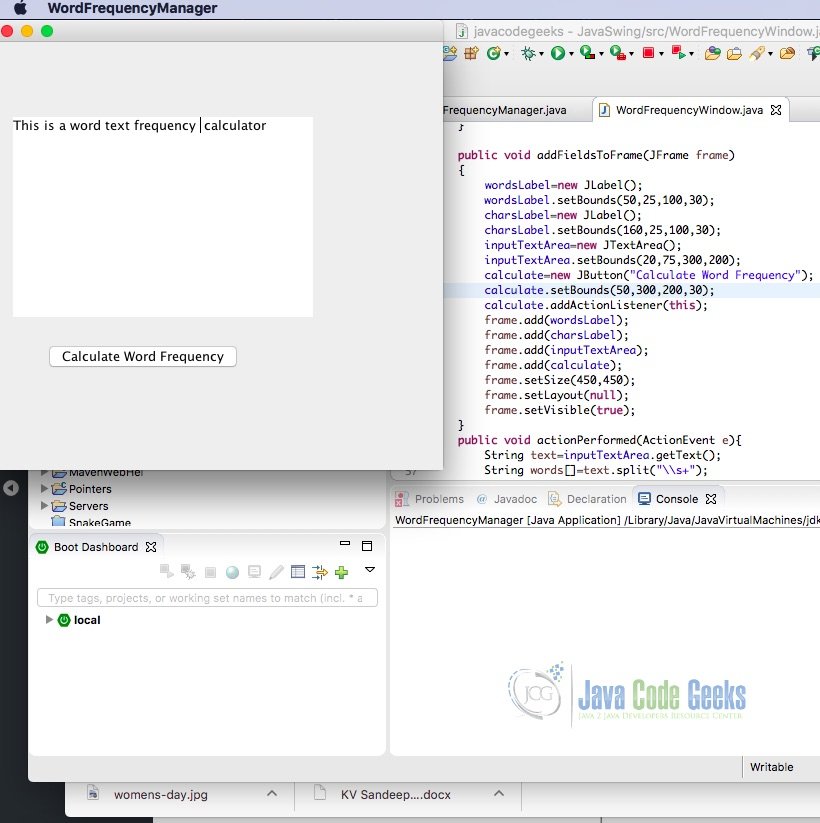
The user clicks on the calculate button to find the words and characters on the screen. The output screen after the calculation is shown in the screenshot below.
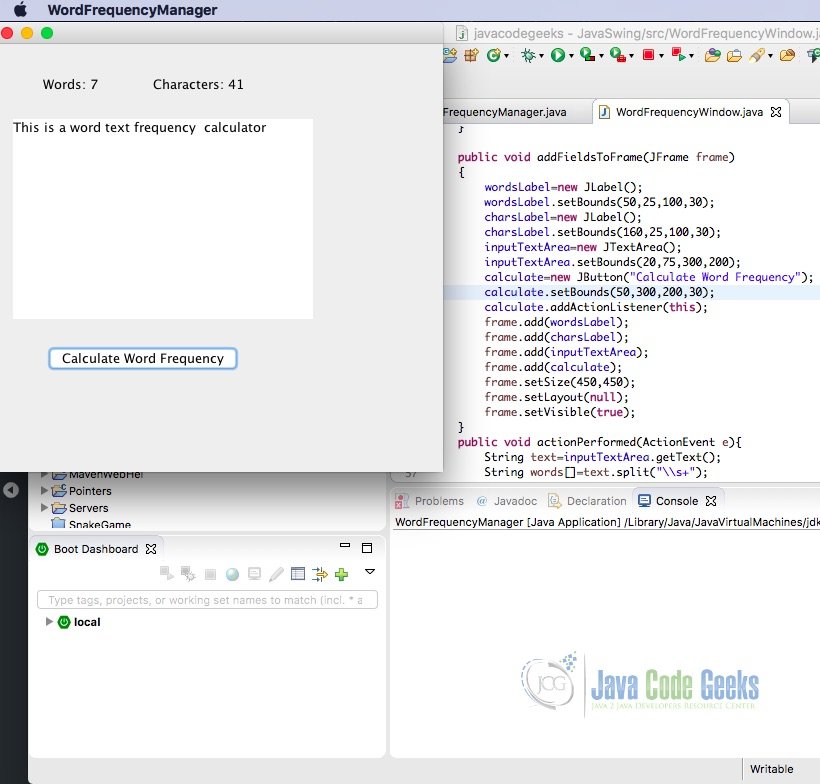
6. Summary
In this tutorial, we saw Java Swing which is a lightweight GUI toolkit that includes a rich set of widgets.
We used Eclipse IDE to create a GUI, we learned what is the AWT Event-Handling and what is the Java Swing class hierarchy. Finally, we saw an advanced Swing Example creating a WordFrequency Application.
7. Download The Source Code
That was a tutorial about how to create a Java Gui with Swing.
You can download the full source code of this example here: How to Create a Java Gui with Swing
Last updated on Mar. 09th, 2020