java.util.concurrent.Semaphore – Semaphore Java Example
In this example, we will show you how to make use of the Semaphore – java.util.concurrent.Semaphore
Class in Java.
1. What is a SEMAPHORE?
A semaphore
in Computer Science is analogous to a Guard at the entrance of a building. However, this guard takes into account the number of people already entered the building. At any given time, there can be only a fixed amount of people inside the building. When a person leaves the building, the guard allows a new person to enter the building. In the computer science realm, this sort of guard is called a Semaphore
. The concept of Semaphore was invented by Edsger Dijkstra in 1965.
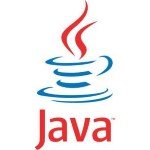
The semaphores in computer science can be broadly classified as :
- Binary Semaphore
- Counting Semaphore
Binary Semaphore has only two states on and off(lock/unlock).(can be implemented in Java by initializing Semaphore to size 1.)
The java.util.concurrent.Semaphore
Class implements a counting Semaphore
.
2. Features of Semaphore Class in Java
- The
Semaphore
Class constructor takes aint
parameter, which represents the number of virtual permits the corresponding semaphore object will allow. - The semaphore has two main methods that are used to acquire() and release() locks.
- The Semaphore Class also supports Fairness setting by the boolean fair parameter. However, fairness leads to reduced application throughput and as such should be used sparingly.
- The
Semaphore
Class also supports non-blocking methods liketryacquire()
andtryacquire(int permits).
SemaphoreDemo.java
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 | package com.javacodegeeks.examples.concurrent; import java.util.concurrent.Semaphore; /** * @author Chandan Singh *This class demonstrates the use of java.util.concurrent.Semaphore Class */ public class SemaphoreDemo { Semaphore semaphore = new Semaphore( 10 ); public void printLock() { try { semaphore.acquire(); System.out.println( "Locks acquired" ); System.out.println( "Locks remaining >> " +semaphore.availablePermits()); } catch (InterruptedException ie) { ie.printStackTrace(); } finally { semaphore.release(); System.out.println( "Locks Released" ); } } public static void main(String[] args) { final SemaphoreDemo semaphoreDemo = new SemaphoreDemo(); Thread thread = new Thread(){ @Override public void run() { semaphoreDemo.printLock(); }}; thread.start(); } } |
Output:
1 2 3 | Locks acquired Locks remaining >> 9 Locks Released |
3. Applications
One of the major application of Semaphore
is while the creation of pooled resources like Database connections.
4. Download the Source Code
We have studied what semaphores are in Java, and how we can use them in our programs.
You can download the source code of this example here: java.util.concurrent.Semaphore – Semaphore Java Example
Last updated on June 01st, 2020