java.lang.NumberFormatException – How to solve Java NumberFormatException
In this example we are going to talk about how to solve the java.lang.numberformatexception . NumberFormatException
in java is discussed in this article. This exception can occur in java applications, web applications, and mobile applications. This can happen in Java, JSP, Servlet, and Android code. This is a common error or failure which happens in an application. It is an unchecked exception. Unchecked Exception is not caught during compile time checking. This exception need not be declared in the method’s signature. It is a run time exception and validation is required by the developer to handle this error. The other common failures are NullPointerException and NoClassDefFoundError.
The class diagram below shows the catch numberformatexception Class hierarchy.
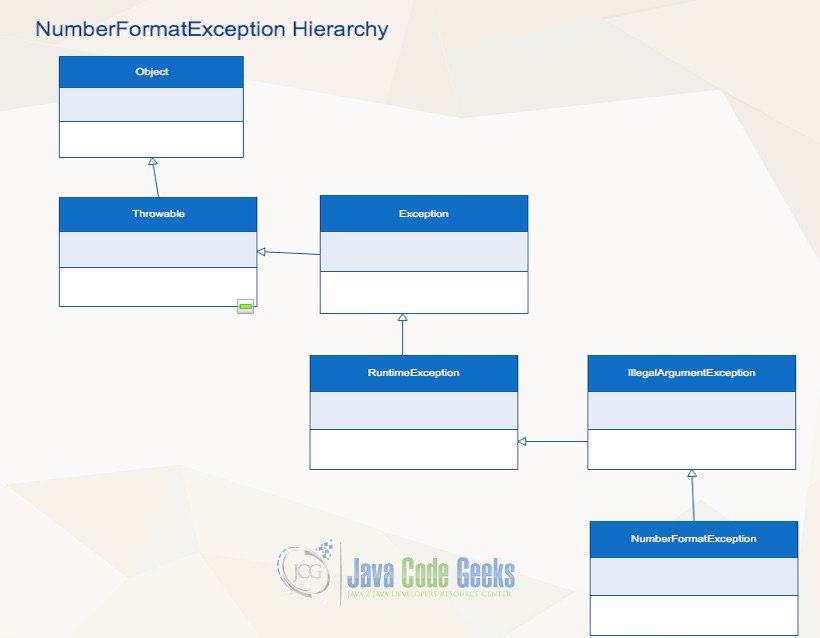
Catch numberformatexception happens while converting a String into different data types such as integer, float, double, long, short, and byte types. The methods below are related to the conversion are :
Data Type | Method |
int | Integer.parseInt() |
float | Float.parseFloat() |
double | Double.parseDouble() |
long | Long.parseLong() |
short | Short.parseShort() |
byte | Byte.parseByte() |
Different reasons for NumberFormatException
are listed below:
- String to be converted is null
- The length of the string is zero
- The string has no numeric characters
- The value of the string does not represent an Integer
- The string can be empty
- It can have trailing space
- It can have a leading space
- The string can be alphanumeric
- The string value might be out of the data type supported range
- The string value and the method used for conversion might be for different data types.
The sequence diagram below shows the possible scenario when a NumberFormatException
can happen.
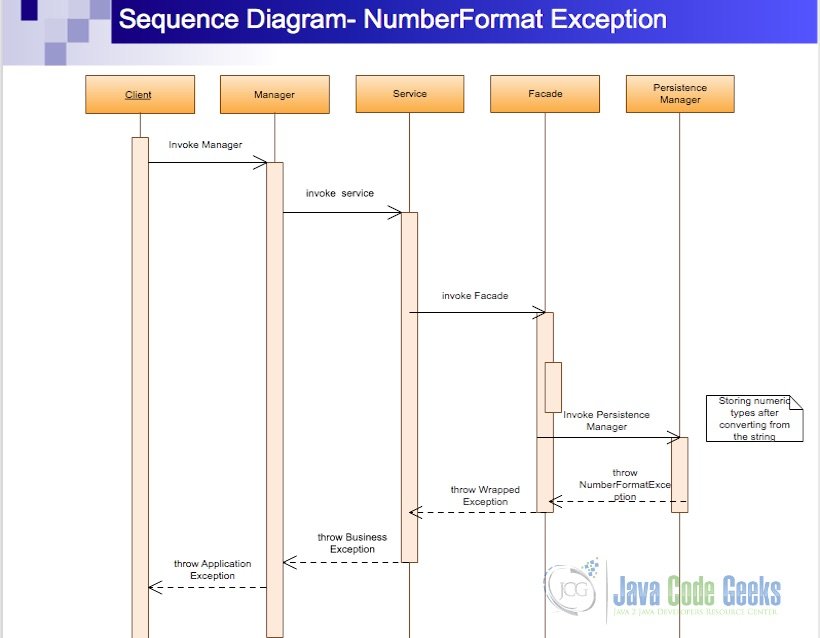
Integer
class has the methods shown below, which can throw NumberFormatException
.
Integer(String s)
– Constructordecode(String s)
parseInt(String s)
parseInt(String s, int radix)
valueOf(String s)
valueOf(String s, int radix)
Below are the code examples showing the conversion of string to other data types such as int, float, double, long, short, and byte.
1.Prerequisites
Java 7 or 8 is required on the linux, windows or mac operating system.
2. Download
You can download Java 7 from Oracle site. On the other hand, You can use Java 8. Java 8 can be downloaded from the Oracle web site .
3. Setup
You can set the environment variables for JAVA_HOME and PATH. They can be set as shown below:
Setup
JAVA_HOME="/desktop/jdk1.8.0_73" export JAVA_HOME PATH=$JAVA_HOME/bin:$PATH export PATH
4. String Conversion to Int
Here is a simple program that will attempt to convert a String to an int. The code below is converting a string of value 0.78 to an Integer.
String to Int
public class StringIntegerConversionExample { public static void main(String[] args) { int value = Integer.parseInt("0.78"); System.out.println(value); } }
The above code is executed by using the command below:
StringIntegerConversionExample
javac StringIntegerConversionExample.java java StringIntegerConversionExample
The output is shown below in the attached screen.
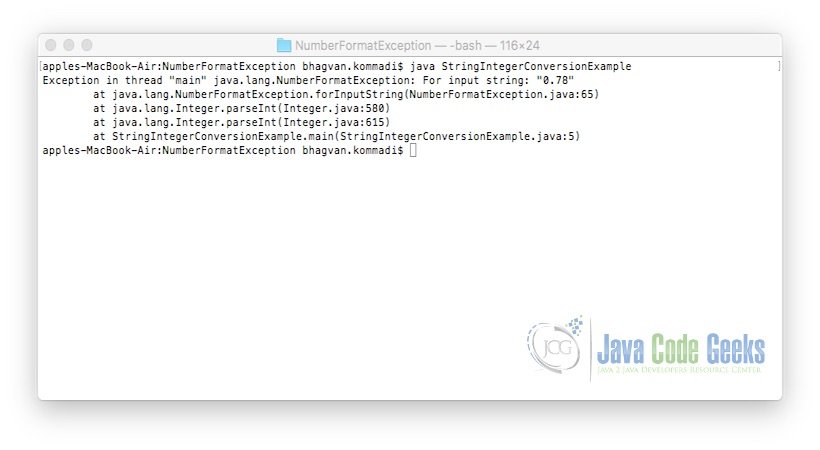
You can change the value to “78”. The code is shown below
String to Int
public class StringIntegerConversionExample { public static void main(String[] args) { int value = Integer.parseInt("78"); System.out.println(value); } }
The above code is executed by using the command below:
StringIntegerConversionExample
javac StringIntegerConversionExample.java java StringIntegerConversionExample
The output is shown below in the attached screen.
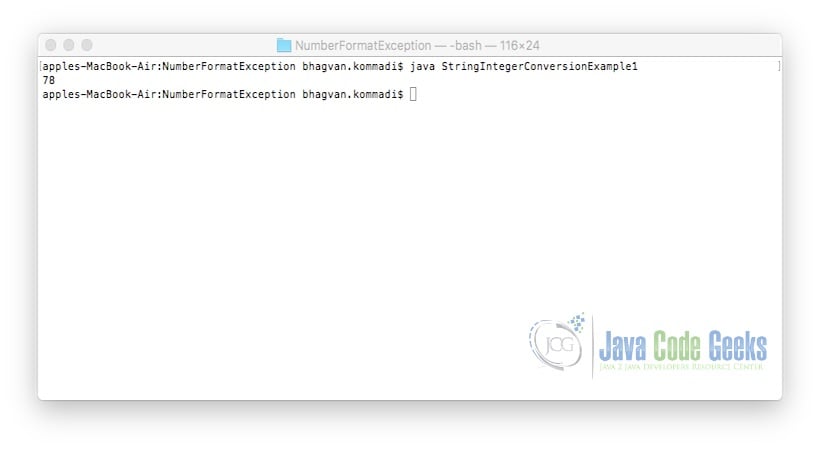
You can change the value to “check”. The code is shown below after the change
String to Int
public class StringIntegerConversionExample { public static void main(String[] args) { Integer.parseInt("check"); } }
The above code is executed by using the command below:
StringIntegerConversionExample
javac StringIntegerConversionExample.java java StringIntegerConversionExample
The output is shown below in the attached screen.
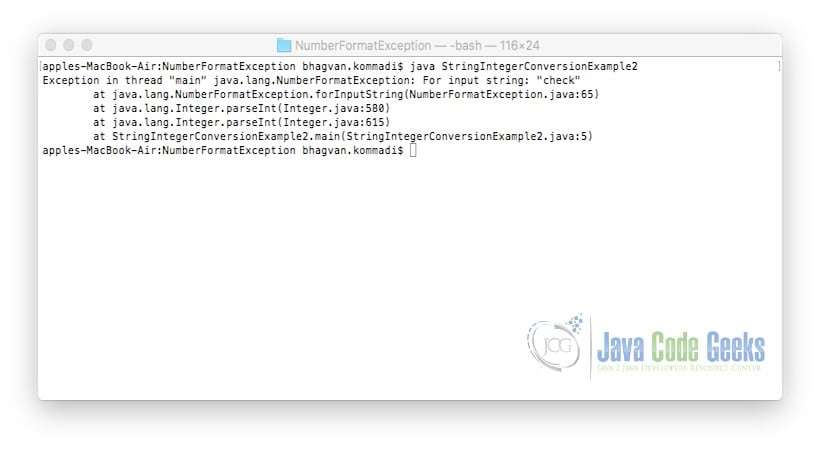
5. String Conversion to Float
Here is a simple program that will attempt to convert a String to a float. The code below is converting a string of value “798!89” to a Float.
String to Float
public class StringFloatConversionExample { public static void main(String[] args) { Float.parseFloat("798!89"); } }
The above code is executed by using the command below:
StringFloatConversionExample
javac StringFloatConversionErrorExample.java java StringFloatConversionExample
The output is shown below in the attached screen.
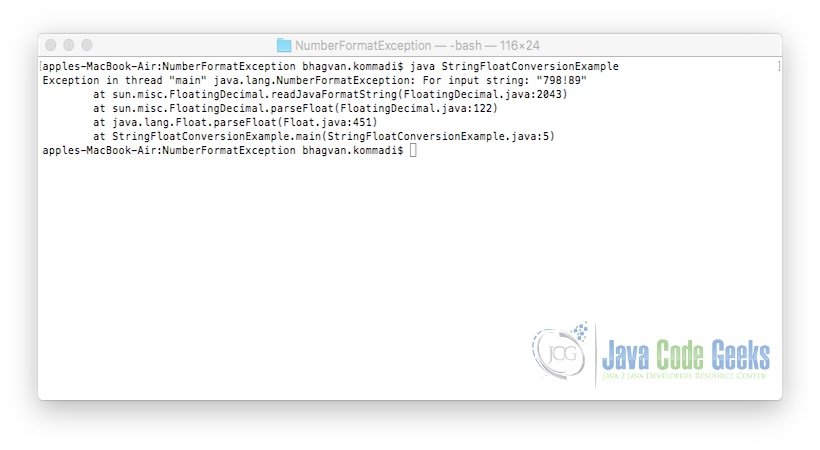
You can change the value to “798.89”. The code is shown below
String to Float
public class StringFloatConversionExample { public static void main(String[] args) { float value = Float.parseFloat("798.89"); System.out.println(value); } }
The above code is executed by using the command below:
StringFloatConversionWorkingExample
javac StringFloatConversionExample.java java StringFloatConversionExample
The output is shown below in the attached screen.
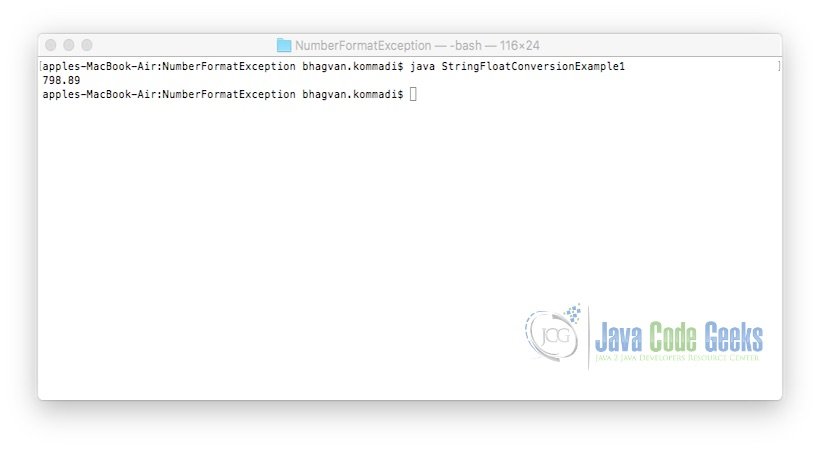
6. String Conversion to Double
Here is a simple program that will attempt to convert a String to a double. The code below is converting a string of value “two.two” to a double.
String to Double
public class StringDoubleConversionExample { public static void main(String[] args) { double aDoublePrim = Double.parseDouble("two.two"); } }
The above code is executed by using the command below:
StringDoubleConversionErrorExample
javac StringDoubleConversionExample.java java StringDoubleConversionExample
The output is shown below in the attached screen.
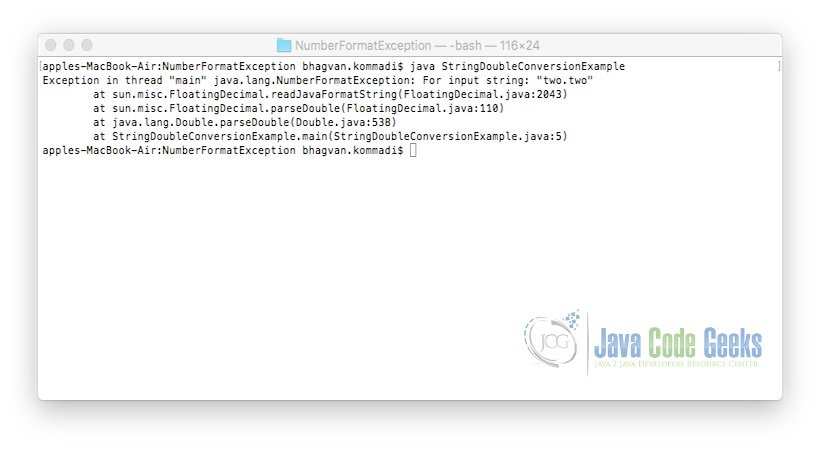
You can change the value to “2.2”. The code is shown below:
String to Double
public class StringDoubleConversionExample { public static void main(String[] args) { double value = Double.parseDouble("2.2"); System.out.println(value); } }
The above code is executed by using the command below:
StringDoubleConversionExample
javac StringDoubleConversionExample.java java StringDoubleConversionExample
The output is shown below in the attached screen.
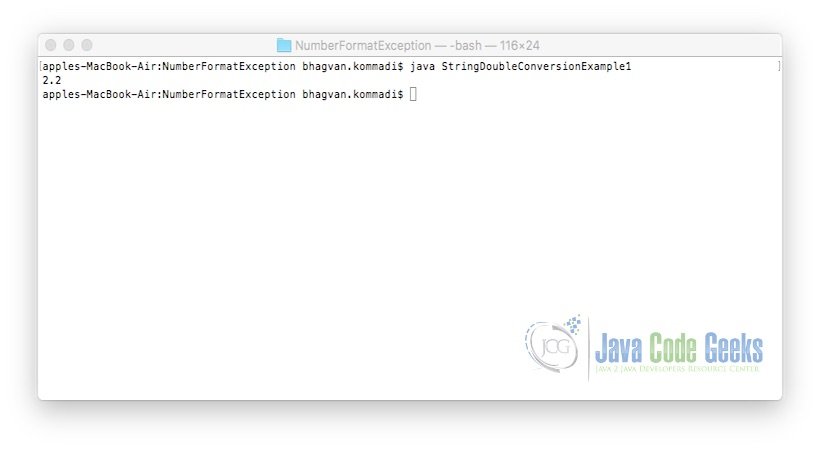
7. String Conversion to Long
Here is a simple program that will attempt to convert a String to a long. The code below is converting a string of value “64403L” to a long.
String to Long
public class StringLongConversionExample { public static void main(String[] args) { Long decodedLong = Long.decode("64403L"); } }
The above code is executed by using the command below:
StringLongConversionErrorExample
javac StringLongConversionExample.java java StringLongConversionExample
The output is shown below in the attached screen.
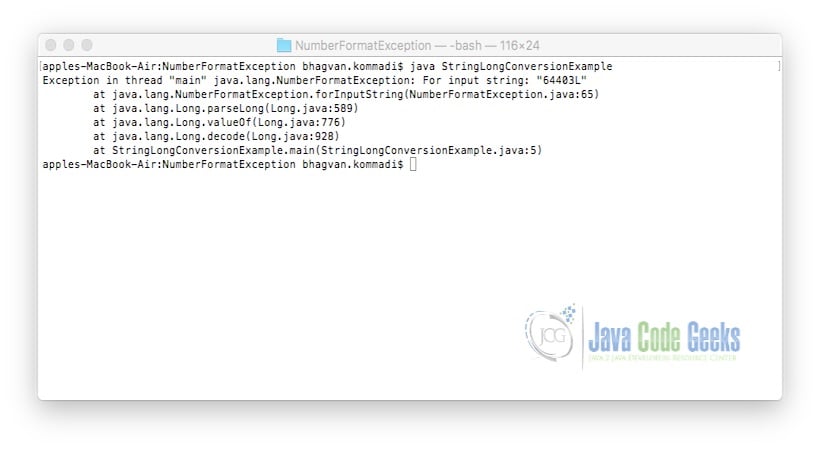
You can change the value to “64403”. The code is shown below:
String to Long
public class StringLongConversionExample { public static void main(String[] args) { Long value = Long.decode("64403"); System.out.println(value); } }
The above code is executed by using the command below:
StringLongConversionExample
javac StringLongConversionExample.java java StringLongConversionExample
The output is shown below in the attached screen.
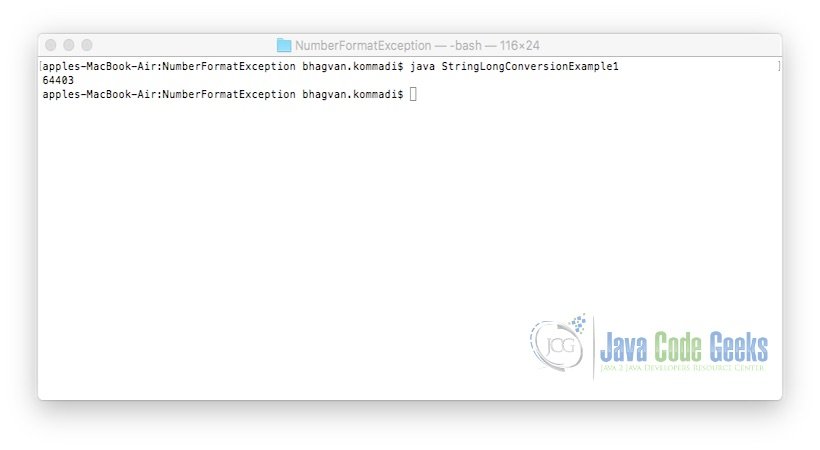
8. String Conversion to Short
Here is a simple program that will attempt to convert a String to a short. The code below is converting a string of value “2 ” to a short.
String to Short
public class StringShortConversionExample { public static void main(String[] args) { Short shortInt = new Short("2 "); } }
The above code is executed by using the command below:
StringShortConversionErrorExample
javac StringShortConversionExample.java java StringShortConversionExample
The output is shown below in the attached screen.
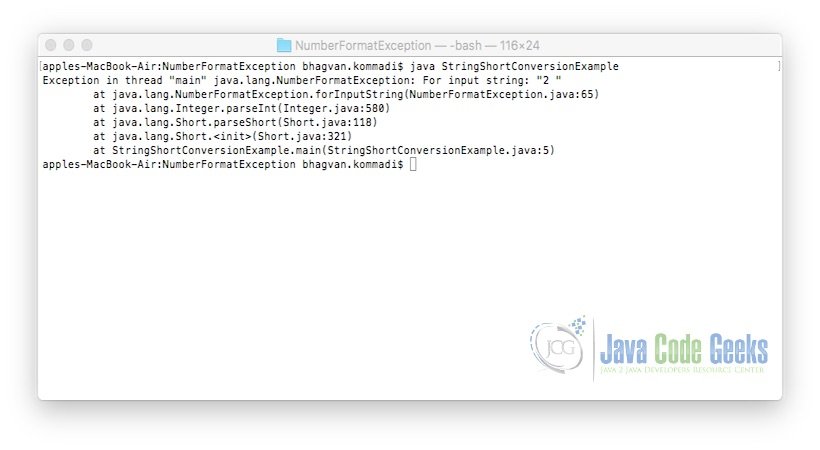
You can change the value to “2” (trimming the spaces). The code is shown below:
String to Short
public class StringShortConversionExample { public static void main(String[] args) { Short shortInt = new Short("2 ".trim()); } }
The above code is executed by using the command below:
StringShortConversionExample
javac StringShortConversionExample.java java StringShortConversionExample
The output is shown below in the attached screen.
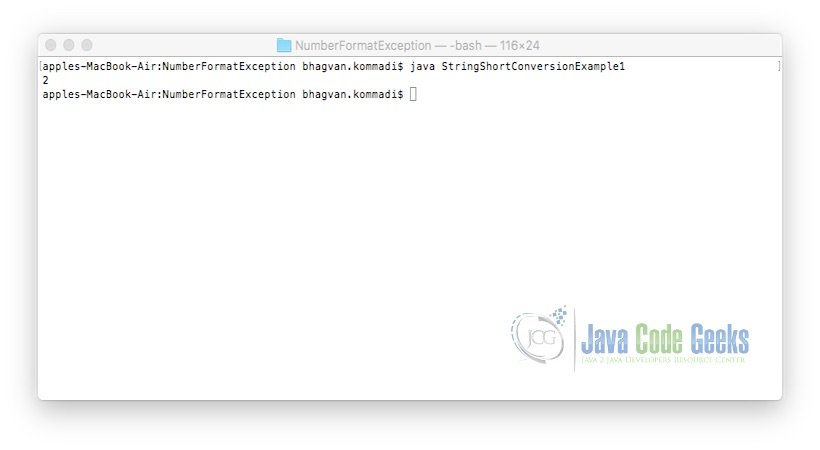
9. String Conversion to Byte
Here is a simple program that will attempt to convert a String to a byte. The code below is converting a string of value “200” to a byte.
String to Byte
public class StringByteConversionExample { public static void main(String[] args) { Byte.parseByte("200"); } }
The above code is executed by using the command below:
StringByteConversionErrorExample
javac StringByteConversionExample.java java StringByteConversionExample
The output is shown below in the attached screen.
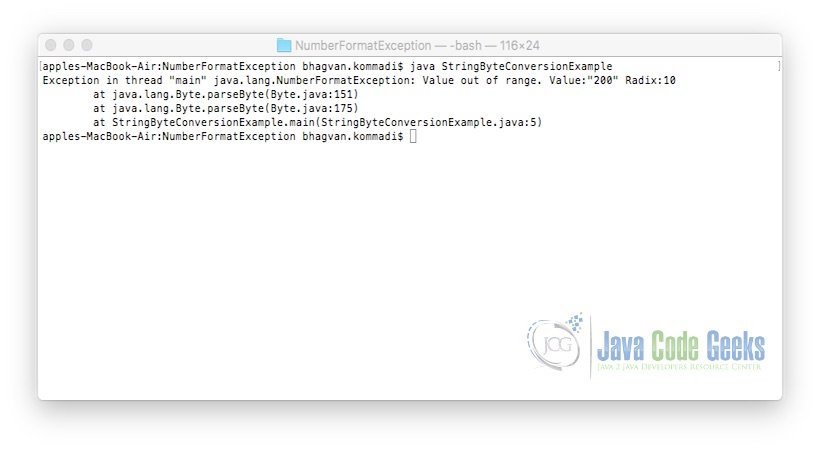
You can change the value to “20” (within the range of -128 to 127). The code is shown below:
String to Byte
public class StringByteConversionExample { public static void main(String[] args) { Byte.parseByte("20"); } }
The above code is executed by using the command below:
StringByteConversionExample
javac StringByteConversionExample.java java StringByteConversionExample
The output is shown below in the attached screen.
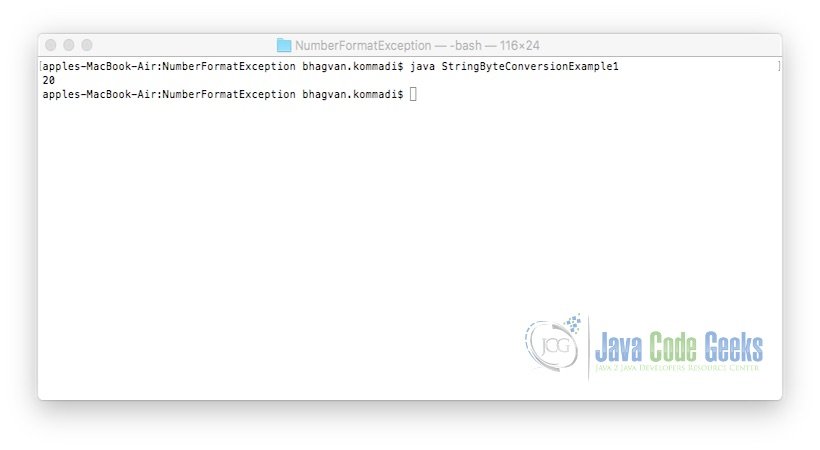
10. How to solve the java.lang.NumberFormatException
NumberFormatException
is thrown when a string is converted to a numeric value. The string format needs to be in the right format of the numeric type. It will fail if the format is wrong. The first step is to check the string for null value. You should trim the blank spaces of the string. If there are multiple numbers with a delimiter space, split the strings.
In any case, the NumberFormatException
is handled by using try catch finally block.
Handling NumberFormatException
public class NumberFormatExceptionHandlingExample { public static void main(String[] args) { try { float value = Float.valueOf("978!79); System.out.println("Parsed value is"+value); } catch(NumberFormatException exception) { System.err.println("Wrong float value"); } finally { } } }
The above code is executed by using the command below:
NumberFormatExceptionHandlingExample
javac NumberFormatExceptionHandlingExample.java java NumberFormatExceptionHandlingExample
The output is shown below the numberformatexception java in the attached screen.
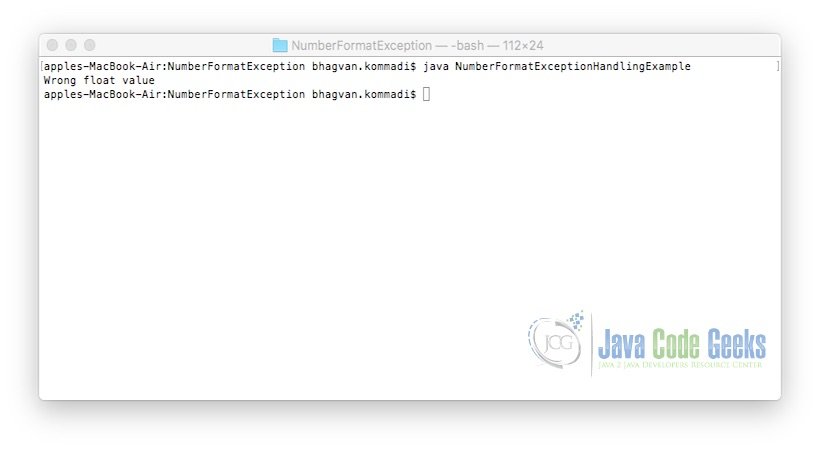
11. Download the Source Code
You can download the full source code of this example here: java.lang.NumberFormatException – How to solve Java NumberFormatException
Last updated on Oct. 07, 2019
java.lang.NumberFormatException: Value out of range, solution for this?
Change datatype from int or float to long. your error will be solved.