Java Strategy Design Pattern Example
1. Introduction to Java Strategy Design Pattern
In this article, we will elaborate java Strategy design pattern in detail which is one of the important behavioral design pattern. The Strategy design pattern is also referred to as a policy pattern which allows the selection of an algorithm at runtime as needed. We define several algorithms or strategies in the java strategy design pattern and choose one by the user as per his or her requirement. This algorithm is transmitted to the processing units as a parameter.
Let us take the example of a web application developer to explain the strategy design pattern. I may simply ask a web developer to write a part of the program for the client to create a user interface if the front end framework is not an problem. One developer has chosen Angular, so with Angular, he will develop the UI. The other developer decides to use React Js to develop the UI for the client in the meantime.And i don’t mind, I have left the details on how developers can write the code to develop the UI for the web application and both have their own strategies. At every step, if the developer feels they need to change thier framework, they can change their strategy and choose to use another framework for the development.So, the startegy design pattern is all about behaviors that change dynamically.
The article begins by explaining the different design patterns available, which we understand with a real life example the Strategy design pattern. This helps to know exactly when you will use the java strategy design pattern. Then after, we will understand how you should precisely program classes to implement a strategy design pattern.
2. Types of Java Design Patterns
Three important briefings are undertaken below of these design patterns. The following sections explain how each pattern has to work with a defined set of rules and how they clean and structured your code.
- Creational patterns
- Structural patterns
- Behavioral patterns
2.1 Creational Pattern
For class instantiation(Object Creation) creational design patterns are used. They make the creation process more dynamic and flexible. Creational patterns in particular can give considerable flexibility in the way objects are created, and initialized. This pattern can be further classified according to creational patterns of class and objects. While class creational models effectively use inheritance in the instantiation process, delegation is used as object creation patterns to adapt the task. Creational Design patterns are divided into six different design types.
2.2 Structural Pattern
Structural Pattern characterizes seven different pattern types. Structural design patterns are the design patterns which simplify design by acknowledging a simple way of interpreting relationships between objects. These design patterns primarily relate classes and objects to form larger structures. The structural design patterns simplify or optimize the structure by understanding the links between structural elements.
2.3 Behavioral Pattern
Behavioral design patterns provide 11 different types of patterns for classifying the method of communication between objects. Behavioral design pattern discusses how objects are communicating. These design pattern defines how various objects and classes propagate or transmit messages into interactions. Behavioural patterns focus on solving issues and distributing duties among objects. These patterns are about communication rather than design.
So, upto now we have gone through the various types of design patterns that are available. Now we will understand one of the behavioral design pattern – Strategy design pattern in detail. The strategy pattern will be used to select the algorithm we want to use during operation. The strategy pattern would be a good use if files were stored in different formats and different sorting algorithms or file compression were executed.
3. Strategy Design Pattern – Real life example
In this section, we will discuss the real world example of Strategy design pattern and try to understand this pattern in a very simple manner. The below image shows that someone wants to travel form his or her house to the airpot to take the flight using various types of modes of transportation that are available.
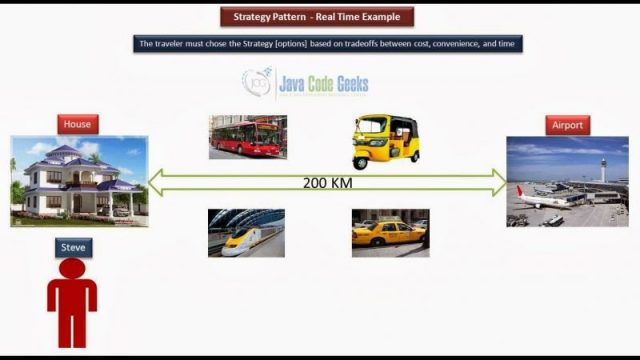
In the above image you can see that on the left side there is a House and on the right side there is an Airpot. Assume that Steve wants to go or travel from the House to the Airpot via some transportation. And the travel distance between the House and the Airpot is 200 hundred kilometer. There are various types of transportation modes Steve can use to go to Airport are available. So, steve can use bus or car or train or taxi to go to the Airport. Steve has four option of transportation to reach airport but he has to choose one from the available four option.
According to the Strategy design pattern for a particular problem there are multiple solution for that problem. From these solutions we have to choose one solution at the runtime. Comparing this with the real life example Steve has four option of transportation and form these four options steve has to choose one option based on the cost, convenience and time to reach the airport. To understand the Strategy design parttern this real world example may be the good example.
4. Implementing Strategy Design Pattern
In this section we will implement the above discussed real world example of travelling using one of the transportation modes in java program. Here, we will consider that someone has to travel to the railway station by one of the transportation mode form the available modes of transportation.
TravelToRailwayStation.java
public interface TravelToRailwayStation { public void gotoRailwayStation(); }
In the above code, we have created TravelToRailwayStation interface in which we have defined a gotoRailwayStation() method.
TravelByAuto.java
public class TravelByAuto implements TravelToRailwayStation { public void gotoRailwayStation() { System.out.println("Karan is Traveling to the Railway Station by an Auto and will be charged 350 Rs"); } }
In the above code, we have created a class called TravelByAuto which implements the TravelToRailwayStation interface. In this class we implemented the gotoRailwayStation() method that we have defined in the TravelToRailwayStation interface.
TravelByBus.java
public class TravelByBus implements TravelToRailwayStation { public void gotoRailwayStation() { System.out.println("Karan is Traveling to the Railway Station by a Bus and will be charged 120 Rs"); } }
In the above code, we have created a class called TravelByBus which implements the TravelToRailwayStation interface. In this class we implemented the gotoRailwayStation() method that we have defined in the TravelToRailwayStation interface.
TravelByTaxi.java
public class TravelByTaxi implements TravelToRailwayStation { public void gotoRailwayStation() { System.out.println("Karan is Traveling to the Railway Station by a Taxi and will be charged 450 Rs"); } }
In the above code, we have created a class called TravelByTaxi which implements the TravelToRailwayStation interface. In this class we implemented the gotoRailwayStation() method that we have defined in the TravelToRailwayStation interface.
Travel.java
public class Travel { private TravelToRailwayStation travelToRailwayStation; public void setTravelToRailwayStation(TravelToRailwayStation railwayStation) { this.travelToRailwayStation = railwayStation; } public TravelToRailwayStation getTravelToRailwayStation() { return travelToRailwayStation; } public void gotoRailwayStation() { travelToRailwayStation.gotoRailwayStation(); } }
In the above code, we have created a class Travel in which we have created a private variable of TravelToRailwayStation interface is travelToRailwayStation. Then we have difined the setters and getters method such as setTravelToRailwayStation() and getTravelToRailwayStation(). And we implemented the gotoRailwayStation() method.
StrategyDesignPattern.java
import java.util.Scanner; public class StrategyDesignPattern { public static void main( String[] args ) { System.out.println("Please enter mode of Transportation Type : 'Bus' or 'Taxi' or 'Auto' "); Scanner scanner = new Scanner(System.in); String transportationType = scanner.next(); System.out.println("Transportation type is : " + transportationType); Travel travel = null; travel = new Travel(); if( "Bus".equalsIgnoreCase(transportationType) ) { travel.setTravelToRailwayStation(new TravelByBus()); } else if("Taxi".equalsIgnoreCase(transportationType)) { travel.setTravelToRailwayStation(new TravelByTaxi()); } else if("Auto".equalsIgnoreCase(transportationType)) { travel.setTravelToRailwayStation(new TravelByAuto()); } System.out.println("Mode of Transportation has : "+ travel.getTravelToRailwayStation()); travel.gotoRailwayStation(); } }
In the above code, we have created class called StrategyDesignPattern inside this class we have created the main() method. In the main() method we created the object of Scanner class to take the input from the user and created a object of Travel class that is travel. At the end we implemented the setters and getters method that we defined in the Travel class.
Please enter mode of Transportation Type : 'Bus' or 'Taxi' or 'Auto' Bus Transportation type is : Bus Mode of Transportation has : TravelByBus@55f96302 Karan is Traveling to the Railway Station by a Bus and will be charged 120 Rs Please enter mode of Transportation Type : 'Bus' or 'Taxi' or 'Auto' Taxi Transportation type is : Taxi Mode of Transportation has : TravelByTaxi@55f96302 Karan is Traveling to the Railway Station by a Taxi and will be charged 450 Rs Please enter mode of Transportation Type : 'Bus' or 'Taxi' or 'Auto' Auto Transportation type is : Auto Mode of Transportation has : TravelByAuto@55f96302 Karan is Traveling to the Railway Station by an Auto and will be charged 350 Rs
From the output we can say that if the traveler have choosen Bus as the mode of transportation then he has to pay 120 Rs. if the traveler choose Taxi then he has to pay 450 Rs. similarly if the traveler choose Auto then he has to pay 350 Rs. to reach to the Railway station. The strategy model offers a way to describe and interchange a family of algorithms, encapsulate each of them as a single object.
5. Benefits of Strategy Design Pattern
Until now, we discussed the Strategy design pattern, the implementation of the Strategy design pattern using Java code and understand the real world example of Strategy design pattern. Let us now discuss some of their advantages. The main advantages of Strategy design pattern are as follows:
- The strategy design pattern is very similar to that of state design pattern. One of the differences is that Context contains the state as instance variable and multiple tasks may be implemented by the state, whereas the strategic design strategy is passed to the method as argument and context object has no variable to store it.
- New algorithms that cooperate with the same interface can easily be introduced by encapsulating the algorithm separately.
- When we have multiple algorithms for certain purposes, strategy design pattern is useful and we want our app to be flexible in selecting any of the algorithms for specific tasks.
- Strategy design pattern allows user to select the algorithm needed without a “Switch” statement or a number of “if- else” statements.
6. Conclusion
This all concerns the strategy design pattern and can also be deliberately shared between the different context objects with the same strategy object. The common objective of the Strategy design pattern, however, should not keep states informed. Simultaneously, all the strategies for choosing the right situation and according to the requirements must be alerted to the application. Also this pattern defines a set of associated algorithms and enables the client to select an algorithm at the latest.
7. Download the Project
You can download the project Folder for the above example from the link below:
You can download the full source code of this example here: Java Strategy Design Pattern Example