Ceasar Cipher Java Example
In this post, we feature a comprehensive Caesar Cipher Java Example and ceaser shift cypher example.
1. What is Caeser Cipher?
The Caesar cipher is one of the earliest known and simplest ciphers. It is a type of substitution cipher in which each letter in the plaintext is shifted a certain number of places down the alphabet. For example, with a shift of 1, A would be replaced by B, B would become C, and so on. The method is named after Julius Caesar, who apparently used it to communicate with his generals.
For Example,
Let plainText, text to be encrypted be, quick brown fox jumps over the lazy dog
.
When we apply caesar cipher over the plain text, with a shift of 1 character, the ciphertext will be, rvjdl cspxo gpy kvnqt pwfs uif mbaz eph
.
Let’s try to understand it character by character,
q with a shift of 1 becomes r.
u with a shift of 1 becomes v.
so on and so forth.
Once we finished encrypting all the alphabets we get to rvjdl cspxo gpy kvnqt pwfs uif mbaz eph
. In this particular example, we have ignored the spaces, for encryption, between the words so they stayed the same.
Now let’s try to decrypt the encrypted text as rvjdl cspxo gpy kvnqt pwfs uif mbaz eph
. We already know the shift used for encryption is 1. So the letters are shifted by 1 character in plain text to generate the cipherText. So In order to decrypt we need to shift each alphabet in cipherText by 1 in reverse order.
If we go character by character in the cipherText,
r will be shifted by 1 in reverse and become q.
v will be shifted by 1 in reverse and become u.
so on and so forth.
After reverse shifting all the characters in the cipherText, we will generate the original plain text, which will be, quick brown fox jumps over the lazy dog
.
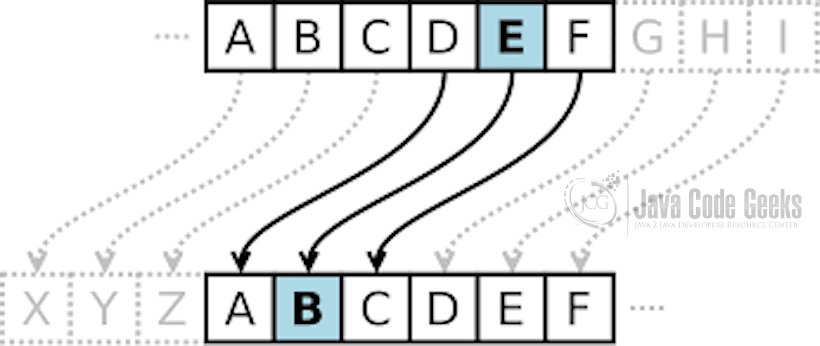
2. How to decrypt the cipher without the key?
There are multiple ways to decrypt a cipher without the key.
One approach would be to use all the possible instances of the keys and applying them on the cipher to generate the plainText. This is a sure shot, albeit, time-consuming process, to generate the plainText from the cipher.
Another technique is more mathematical in nature, widely used by cryptographers, and is called Cryptoanalysis. Cryptanalysis is the study of ciphertext, ciphers, and cryptosystems with the aim of understanding how they work and finding and improving techniques for defeating or weakening them.
3. Implementing Caeser Cipher Java Example
In this section, we will discuss the implementation of Caesar Cipher in java.
The code is shown below in CaesarCipher.java
.
CaesarCipher.java
class CaesarCipher {
// Encrypts text using shift
public static StringBuffer encrypt(String text, int shift) {
StringBuffer result = new StringBuffer();
for (int i = 0; i < text.length(); i++) {
if (Character.isUpperCase(text.charAt(i))) {
char ch = (char) (((int) text.charAt(i) +
shift - 65) % 26 + 65);
result.append(ch);
} else {
char ch = (char) (((int) text.charAt(i) +
shift - 97) % 26 + 97);
result.append(ch);
}
}
return result;
}
// Decrypts cipher using shift
public static StringBuffer decrypt(String cipher, int shift) {
StringBuffer result = new StringBuffer();
for (int i = 0; i < cipher.length(); i++) {
if (Character.isUpperCase(cipher.charAt(i))) {
char ch = (char) (((int) cipher.charAt(i) +
shift - 65) % 26 + 65);
result.append(ch);
} else {
char ch = (char) (((int) cipher.charAt(i) +
shift - 97) % 26 + 97);
result.append(ch);
}
}
return result;
}
public static void main(String[] args) {
String originalText = "ATTACKATONCE";
int shiftCount = 1;
System.out.println("Caesar Cipher Example");
System.out.println("Encryption");
System.out.println("Text : " + originalText);
System.out.println("Shift : " + shiftCount);
String cipher = encrypt(originalText, shiftCount).toString();
System.out.println("Encrypted Cipher: " + cipher);
System.out.println("Decryption");
System.out.println("Encrypted Cipher: " + cipher);
System.out.println("Shift : " + shiftCount);
String decryptedPlainText = decrypt(cipher, 26 - shiftCount).toString();
System.out.println("Decrypted Plain Text : " + decryptedPlainText);
}
}
In the code shown in CaesarCipher.java
, we have defined 2 functions, one for encryption and one for decryption.
In the encryption function, we traverse each character from the input text and we calculate the character which will replace this character by using the shift as,
encrypted_character = (current_character + shift) mod 26
we apply this formula for each character in the input string to generate the encrypted ciphertext.
In the decryption function, we traverse each character from the encrypted text and we calculate the character which will replace this character by using the shift as,
plain_character = (current_character_from_encrypted_string + (26-shift)) mod 26
we apply this formula for each character in the cipherText to generate the decrypted plaintext.
The output for CaesarCipher.java
is shown in the snapshot below,
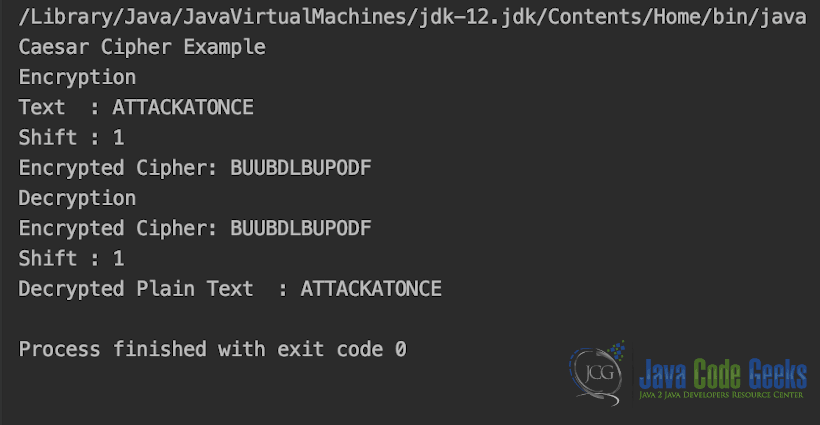
4. Summary
To Summarise what we have covered in this article, we have gathered a basic understanding of the Caeser cipher, which is a kind of substitution cipher. We have covered the implementation detail of the encryption and decryption using Caeser cipher. There are other ciphers such as the Vigenère cipher, that employs Caesar cipher as one element of the encryption process, but details of those are beyond the scope of this article.
5. Download the Source code
That was a Caesar Cipher Java Example.
You can download the full source code of this example here: Caesar Cipher Java Example