C# vs Java: The Most Important Differences
In this post, we feature a comprehensive article about Java vs C# and their most important differences. Java and C# are the object-oriented programming languages. C# and Java are platform-independent languages. In this tutorial, we see the features of Java and C# to see the differences.
1. Overview
We look at the comparison of Java vs C# languages in this article. They can be used for developing software and executing the code. Java code is converted into bytecode after compilation. The java interpreter runs the bytecode and the output is created. James Gosling came up with Java while working in Sun. C# language can be executed on CLR (Common Language Runtime). Anders Hejlsberg was the key person behind C# language development in Microsoft.
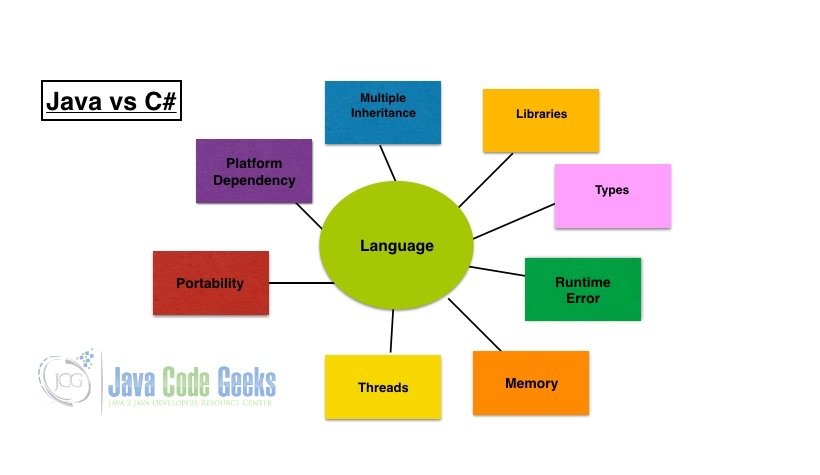
2. C# vs Java
2.1 Prerequisites
Java 8 is required on the linux, windows or mac operating system. Eclipse Oxygen can be used for this example. Visual studio code can be used for C# development.
2.2 Download
You can download Java 8 from the Oracle web site . Eclipse Oxygen can be downloaded from the eclipse web site. Visual Studio code can be downloaded from the link.
2.3 Setup
2.3.1 Java Setup
Below is the setup commands required for the Java Environment.
Java Setup
JAVA_HOME="/desktop/jdk1.8.0_73" export JAVA_HOME PATH=$JAVA_HOME/bin:$PATH export PATH
2.3.2 C# Setup
Visual Studio Code can have a C# extension from the marketplace link.
2.4 IDE
2.4.1 Eclipse Oxygen Setup
The ‘eclipse-java-oxygen-2-macosx-cocoa-x86_64.tar’ can be downloaded from the eclipse website. The tar file is opened by double click. The tar file is unzipped by using the archive utility. After unzipping, you will find the eclipse icon in the folder. You can move the eclipse icon from the folder to applications by dragging the icon.
2.4.2 Visual Studio Code
Run the visual studio installer and update the C# extension from the marketplace.
2.5 Memory Management
Java language has features related to memory management and it is a memory-safe language. Garbage collection is a feature that helps in collecting the resources which are free and released. C# has automatic garbage collection.
2.6 Exceptional Handling
In Java, exception handling is possible by using try, catch and finally blocks. In java, exception classes can be extended and created for various errors. If a method throws an exception in Java, try and catch has to be created to handle the exception while invoking the method. In C#, runtime errors are handled as exceptions. C# has try, catch, finally, and throw blocks to handle exceptions.
2.7 Multiple Inheritance
Multiple inheritance is not supported in Java and C#. Let us take an example to see how it is handled in Java and C#. Truck is an vehicle and a machine.
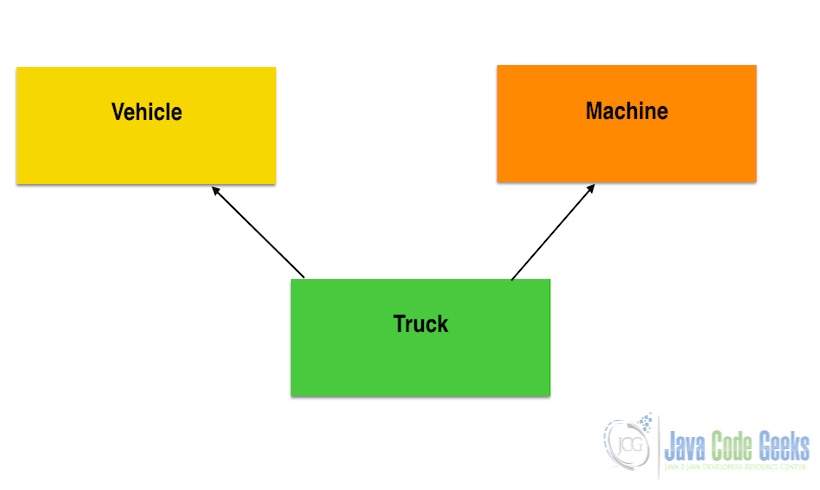
Java does not support multiple inheritance. Each class is able to extend only on one class but is able to implement more than one interface. The sample code shows below Truck
class implementing interfaces Machine
and Vehicle
.
Interfaces in Java
interface Machine { int velocity=50; public int getDistance(); } interface Vehicle { int distanceTravelled=100; public int getVelocity(); } public class Truck implements Machine, Vehicle { int time; int velocity; int distanceTravelled; public Truck(int velocity, int time) { this.velocity = velocity; this.time = time; } public int getDistance() { distanceTravelled= velocity*time; System.out.println("Total Distance is : "+distanceTravelled); return distanceTravelled; } public int getVelocity() { int velocity=distanceTravelled/time; System.out.println("Velocity is : "+ velocity); return velocity; } public static void main(String args[]) { Truck truck = new Truck(50,2); truck.getDistance(); truck.getVelocity(); } }
In C#, we use interfaces to handle multiple inheritance.
Interfaces in C#
using System; interface Machine { public int velocity=50; public int GetDistance(); } interface Vehicle { public int distanceTravelled=100; public int GetVelocity(); } public class Truck : Machine, Vehicle { public int time; public int velocity; public int distanceTravelled; public Truck(int velocity, int time) { this.velocity = velocity; this.time = time; } public int GetDistance() { distanceTravelled= velocity*time; Console.writeLine("Total Distance is : "+distanceTravelled); return distanceTravelled; } public int GetVelocity() { int velocity=distanceTravelled/time; Console.writeLine("Velocity is : "+ velocity); return velocity; } public static void Main(String args[]) { Truck truck = new Truck(50,2); truck.GetDistance(); truck.GetVelocity(); } }
2.8 Threads
Java has in built classes to create threads. To create a new thread, a class has to extend a Thread
class and the run method has to be overridden.
Java Threads
public class NewThread extends Thread { public void run() { System.out.println("Thread running now"); } public static void main(String args[]) { NewThread newThread =new NewThread(); newThread.start(); } }
Java provides another way to create Threads. A class which implements Runnable
can be instantiated and passed as a parameter to the Thread
class. Sample code is provided below:
Java ThreadObject
public class ThreadObject implements Runnable { public void run() { System.out.println("ThreadObject running"); } public static void main(String args[]) { ThreadObject threadObject =new ThreadObject(); Thread thread =new Thread(threadObject); thread.start(); } }
In C#, Thread
class is used for creating threads and executing tasks. Sample code is shown below;
C# Thread
using System; using System.Threading; public class ThreadObject { public static void Main(string[] args) { Thread thread = Thread.CurrentThread; thread.Name = "Thread1"; Console.WriteLine(thread.Name); } }
2.9 Portability
Java language is interpreted by the Java interpreter on the computer independent of the operating system.
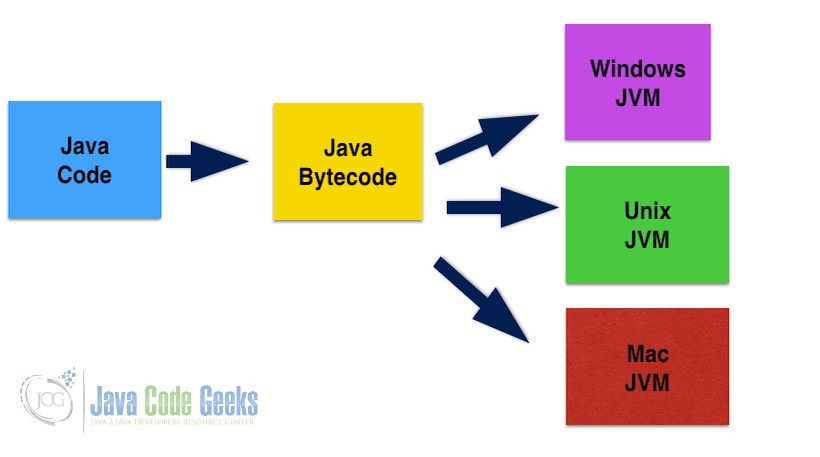
Common Language Runtime in .Net has capability to run code in different .Net languages like C#, VB.Net, C++ and F#. CLR compiles the code into native code which is run on the CPU.
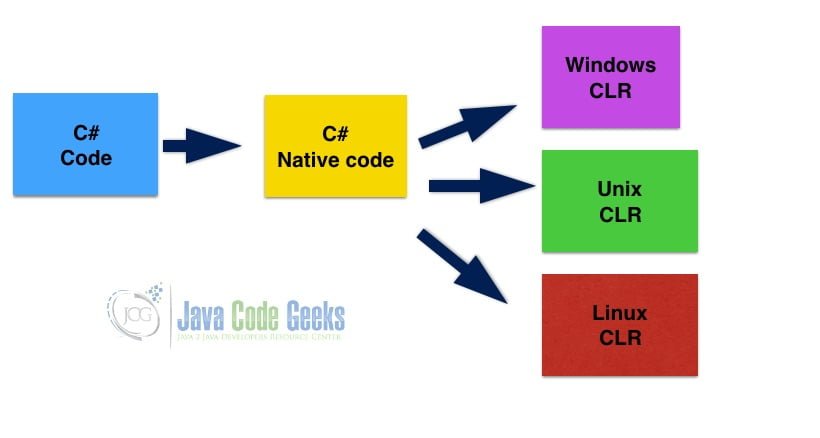
2.10 Types
Java language has primitive and object types. Java has features related to autoboxing which converts the types automatically. The java.lang.Object
class is the base class for all the classes and Java follows the single root chain of command.
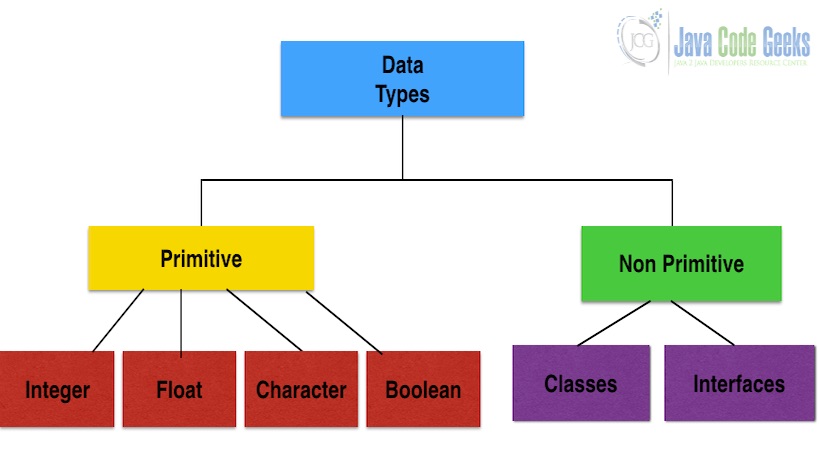
C# Data Types are of two types predefined and user-defined.System.Object
is the base class for all the classes
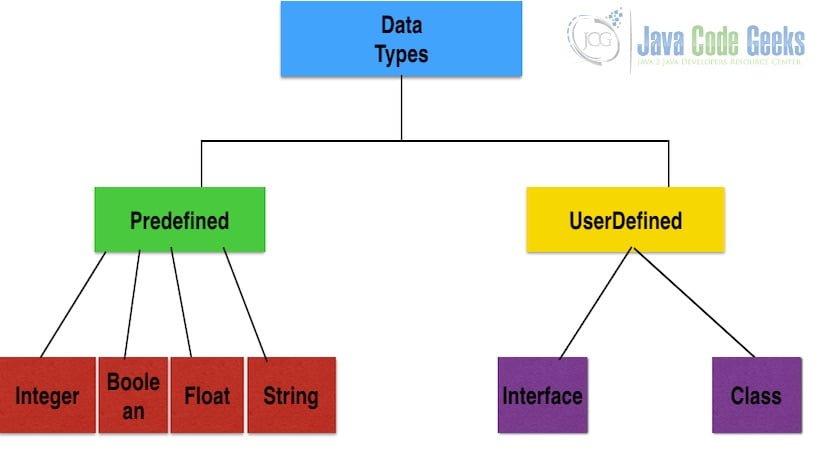
2.11 Libraries
Java packages help in packaging classes. Package scope is another feature in Java language. Java archives help in grouping the package of classes for execution and installation purposes.
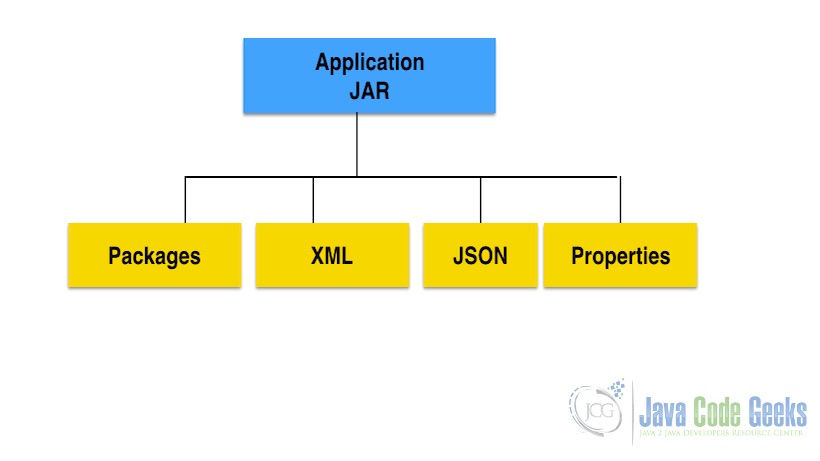
C# libraries can be created which has namespaces, classes, and methods
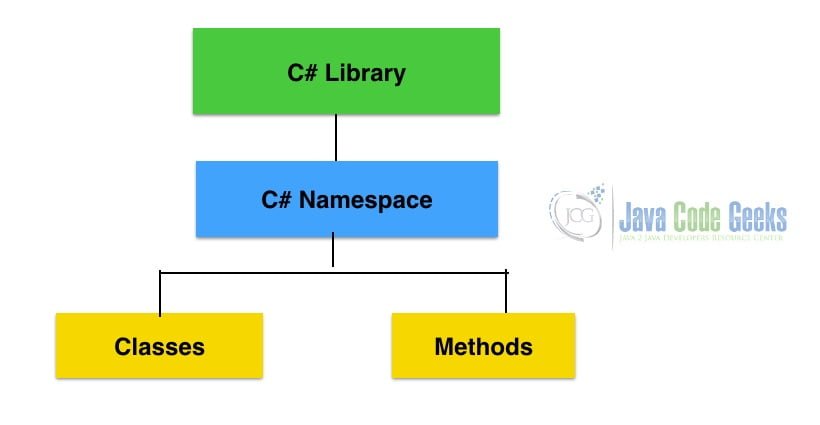
2.12 Runtime Errors
In java, runtime errors are presented by the compiler and the interpreter. C# has support for handling runtime errors in .Net.
2.13 Documentation
Java has the feature to support comments which can be used for documentation generator. C# has XML documentation comments which can be used for documentation.
2.14 Mobile & Web & Desktop
Java language can be used for mobile, web and desktop application development. C# also can be used for developing web, desktop, and mobile applications.
3. Summary
Overall, Java has great benefits over C#. The comparison table below captures the differences between Java and C#.
Comparison Table
Feature | Java | C# |
Memory Management | Garbage collection is a feature in Java.Pointers are not there in Java. Java programs consume more memory compared to C# programs. | C# has automatic garbage collection and pointers. |
Inheritance | Interfaces can be used for multiple inheritance. Single Inheritance is supported in Java. | Single inheritance is supported in C# |
Threads | Java has class Thread and interface Runnable to use threads. | C# has class Thread. |
Portability | Java byte code is platform dependent. | C# code can be compiled to native code which can be executed by CLR. |
Access Control | Encapsulation helps in access control of the class variables and properties in java. | C# has an encapsulation feature and operator overloading. |
Types | A single root chain of command pattern is used in Java. | C# has a single root chain of command. |
Libraries | Java archives are used for building java libraries. | C# libraries are created using namespaces, classes, and methods |
Runtime error | Runtime errors are detected in compilation and execution stages in Java | In C#, runtime errors are handled by exceptions |
Performance | Java performance is better compared to C# | C# is slower compared to java. |
4. Download the Source Code
You can download the full source code of this example here: C# vs Java: The Most Important Differences