ArrayList to Array Java Example
In this tutorial, we will look at various ways to convert ArrayList to array java and we will discuss the efficient approach for it.
1. ArrayList to Array in Java
The first step for our example is to create an ArrayList
List numbers = new ArrayList(); final int endRange = 100000000; IntStream.range(0, endRange).forEach(value -> { numbers.add(value + 1); });
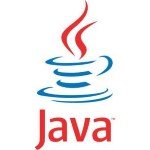
- We are going for a huge range of 100000000 numbers.(Not so huge for modern CPU)
- We iterate the range and add the number to
ArrayList
. - Now an
ArrayList
of size 100000000 has been created.
To measure the time taken for each method, we will leverage a simple StopWatch
class
static class StopWatch { private static long time = 0; static void start() { time = System.currentTimeMillis(); } static long end() { long diff = System.currentTimeMillis() - time; time = 0; return diff; } }
- start – used to start the timer and store the value in time variable
- end – used to return the difference in time(milliseconds) and then reset the timer
1.1 toArray
The simplest approach to convert arraylist to array java is by using toArray
method.
Object[] arr = numbers.toArray(); System.out.println(arr.length);
- Here the
ArrayList
of integers is converted to array of objects. - Since we do not specify type information during cast, it provides the default type of object.
- Internally
ArrayList
maintains only an object array which is copied over to a newly created array using nativeSystem.arraycopy
method
1.2 toArray with Type
Here we are using toArray
method to get the array back in a specified type(Integer)
Integer[] dummy = new Integer[0]; Integer[] ints = numbers.toArray(dummy); System.out.println(ints.length); System.out.println(dummy.length);
- The first step is to create an Array of the destination type.(dummy)
- Here, We have created an array of size 0.
- Once the
toArray
method is called, it checks if the size of array can hold the elements inArrayList
. - If size is lesser, it creates a new array using the above method but converts it to specified type.
- Else, the passed array is populated with the elements from the
ArrayList
. - Running the above code produces the below output
100000000 0
If the dummy is initialized with the actual size of 100000000, then we will get the following output.
100000000 100000000
1.3 Filling the Array
This is the most verbose approach among the three.
int[] intArray = new int[100000000]; for (int i = 0; i < numbers.size(); i++) { intArray[i] = numbers.get(i); } System.out.println(intArray.length);
- This involves iterating the
ArrayList
manually index by index - This also provides greater control to the programmer compared to the other two.
Below is a complete source with all the examples joined together. This uses a size of 10000 to ensure runtime does not fail.
import java.util.ArrayList; import java.util.List; import java.util.stream.IntStream; public class ArrayListDemo { public static void main(String[] args) { List<Integer> numbers = new ArrayList<>(); final int endRange = 10000; IntStream.range(0, endRange).forEach(value -> { numbers.add(value + 1); }); IntStream.range(0, 3).forEach(index -> { switch (index) { case 0: toObjectArray(numbers); break; case 1: toArray(numbers); break; case 2: fillArray(numbers); break; } }); } private static void fillArray(List<Integer> numbers) { int[] intArray = new int[10000]; for (int i = 0; i < numbers.size(); i++) { intArray[i] = numbers.get(i); } System.out.println(intArray.length); } private static void toArray(List<Integer> numbers) { Integer[] dummy = new Integer[0]; Integer[] ints = numbers.toArray(dummy); System.out.println(ints.length); System.out.println(dummy.length); } private static void toObjectArray(List<Integer> numbers) { Object[] arr = numbers.toArray(); System.out.println(arr.length); } }
2. Comparison of Approaches
toArray
involves creating an additional array of the same size as source and it uses System.arraycopy
to copy from source array. It uses space of n and also uses the least execution time.
toArray
with type also uses System.arraycopy
method to copy from source array. But it checks if destination array is of required length but if not creates a new array of ArrayList
size. It involves additional space if the input array is of size k i.e. k+n and sometimes only n. The time complexity also adds slightly due to this condition and also coercing it into the specified type.
The third method has predictable time complexity of O(n). It iterates through all elements and sets the value in a new array. This approach has space complexity of n elements matching the size of source array.
On Average test runs, Approach 3 seems to be slowest with measures as below
Elapsed ms:351 Elapsed ms:593 Elapsed ms:741
But if we do make the code run 100 times and go for average execution time
Elapsed ms:773 Elapsed ms:1029 Elapsed ms:318
The third approach seems to be faster. This is because of the reason that the entire array would be already loaded in memory because of the frequent access and subsequent index access by position will be faster. In other two approaches, it involves copying the entire array into new array every time.
3. Download the Source Code
You can download the full source code of this example here: ArrayList to Array Java Example