Array methods Java Tutorial
In this post, we feature a comprehensive Array methods Java Tutorial.
You can watch the following video and learn how to use arrays in Java:
1. Introduction to Arrays in Java
In java, Arrays can be think of the sequential collection of objects. An array can contain a number of items that are all located next to each other in memory.
If the number of items in an array is zero, then the array is said to be empty. The variables contained in an array have no names; instead, they are referenced by array access expressions that use non-negative integer index values.
These index values will start from zero, hence arrays in java follow a 0-index pattern.
Table Of Contents
- 1. Introduction to Arrays in Java
- 2. Common Operations supported by Arrays in Java
- 3. Updates on Array Methods in Java 8
- 4. Updates on Array Methods in Java 9
- 5. Summary
- 6. Download Source Code
1.1 Create and Initialise an Array
In this section we will learn how to create an array and then how to initialise an array.
ArrayCreateAndInitialise.java
public class ArrayCreateAndInitialise {
public static void main(String[] args) {
// we will create a simple integer array
int[] arrOne;
int[] arrTwo = {};
int arrThree[] = new int[4];
// how to initialise the array
arrOne = new int[]{1, 1, 1, 1};
arrTwo = new int[]{2, 2, 2, 2};
arrThree = new int[]{3, 3, 3, 3};
// to print the content of the array
System.out.println("Printing arrOne elements");
printArray(arrOne);
System.out.println("Printing arrTwo elements");
printArray(arrTwo);
System.out.println("Printing arrThree elements");
printArray(arrThree);
}
public static void printArray(int[] arr){
for (int i = 0; i < arr.length; i++) {
System.out.println(arr[i]);
}
}
}
In the code snippet shown in ArrayCreateAndInitialise.java
, we have created and initialised three arrays showing different mechanisms to create the arrays in java. arrOne
is a variable which will hold the reference to an integer array. arrTwo
is an empty array as shown in the code snippet. If we try to get the size of arrTwo
using arrTwo.length
it will return 0, on the other hand if we try to take the size of the arrOne
using arrOne.length
it will result in compilation error, as arrOne
is not been initialised.
Now if we examine the arrThree
, we can clearly make out that the size of the array arrThree
will be 4 as shown in int arrThree[] = new int[4];
.
Another noteworthy point here is that we have only created all the three arrays till line 6 in ArrayCreateAndInitialise.java
. We have not initialise the arrays as such. Until we specific provide values to be stored in the array, java will put default values in the array in place of each element sort of as a placeholder. These default values will depend on the data type of the array. For our case, since all arrays are of Integer
datatype, java will store 0 value as a placeholder.
From line 9 onward in ArrayCreateAndInitialise.java
we initialise all the arrays and if we try to print the elements of each of the array we will get the output as shown in Fig. 1 below.
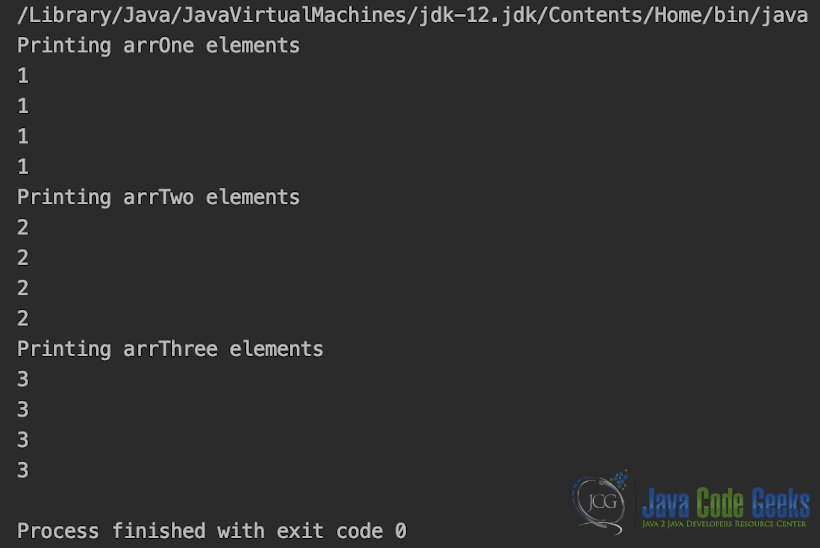
1.2 Accessing Array Elements
In this section we will discuss how to access the array elements. If we look at the code printArray
method in ArrayCreateAndInitialise.java
, we learn that we are actually accessing elements in the array using indexes. We have already discussed, that individual elements in array are stored in sequential order, meaning adjacent to each other, with sequence starting from 0. In essence we can say that we can find first element of arr
array at arr[0]
, second in arr[1]
, so on and so forth.
From this we also get one insight, that since we know that array elements are stored in a sequence, we can directly go to the element which we want. we know that indices starts from 0, so if we want to retrieve 5th element in an array arr
, we can access it directly using arr[4]
. we didn’t have to visit all the previous elements in arr
from index 0 to index 4 to get to arr[4]
. Hence we can conclude that arrays in java supports random access.
2. Common Operations supported by Arrays in Java
In this section we will look at the common operation supported by arrays. The methods to support these operations are provided by Arrays
class of the java.util package
.
2.1 Search
In this section we will look at the methods provided by java for search operations in an array. We will be using these methods to search for a particular element in an array.
2.1.1 static int binarySearch(int[] a, int key)
Searches the specified array for the given value using the binary search algorithm and returns the value.
2.1.2 static int binarySearch(int[] a, int fromIndex, int toIndex, int key)
Searches between the range specified in the specified array for the given value using the binary search algorithm and returns the value.
We will see both search methods in action in code snippet below.
ArraySearch.java
import java.util.Arrays;
public class ArraySearch {
public static void main(String[] args) {
int intArr[] = {10, 20, 15, 22, 35};
Arrays.sort(intArr);
int searchKey = 22;
int secondSearchKey = 15;
System.out.println(searchKey
+ " found at index = "
+ Arrays
.binarySearch(intArr, searchKey));
System.out.println(
secondSearchKey
+ " found at index = "
+ Arrays
.binarySearch(intArr, 1, 3, secondSearchKey));
}
}
In ArraySearch.java
we see both version of binarySearch()
methods in action. Output of ArraySearch.java
can be seen in the Fig.2. below.
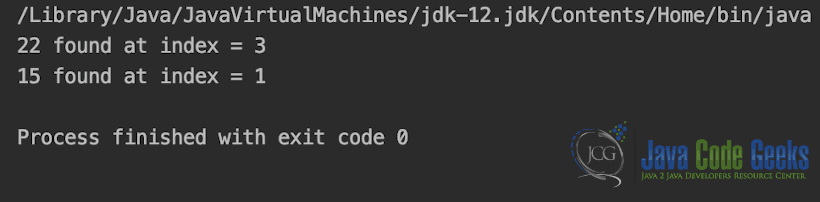
2.2 Sort
In this section we will look at the methods provided by java for sort operations in an array. We will be using these methods to sort an array.
2.2.1 static void sort(int[] a)
Sorts the specified array into ascending order. This is a serial sorting method and works well with small to large arrays.
2.2.2 static void parallelSort(int[] a)
Sorts the specified array into ascending order. This works well with array with huge number of elements.
we will see both sort methods in action in code snippet below.
ArraySort.java
import java.util.Arrays;
public class ArraySort {
public static void main(String[] args) {
int arrayToBeSorted[] = {10, 20, 15, 22, 35};
System.out.println("Demonstrating normal sort operation");
System.out.println("Unsorted Integer Array: "
+ Arrays.toString(arrayToBeSorted));
Arrays.sort(arrayToBeSorted);
System.out.println();
System.out.println("Sorted Integer Array: "
+ Arrays.toString(arrayToBeSorted));
System.out.println();
System.out.println("Demonstrating parallel sort operation");
System.out.println();
int secondArrayToBeSorted[] = {-10, 20, 0, 15, -22, 35};
System.out.println("Unsorted Integer Array: "
+ Arrays.toString(secondArrayToBeSorted));
Arrays.parallelSort(secondArrayToBeSorted);
System.out.println();
System.out.println("Sorted Integer Array: "
+ Arrays.toString(secondArrayToBeSorted));
}
}
In ArraySort.java
we see Arrays.sort()
and Arrays.parallelSort()
methods in action. Output of ArraySort.java
can be seen in the Fig.3. below.
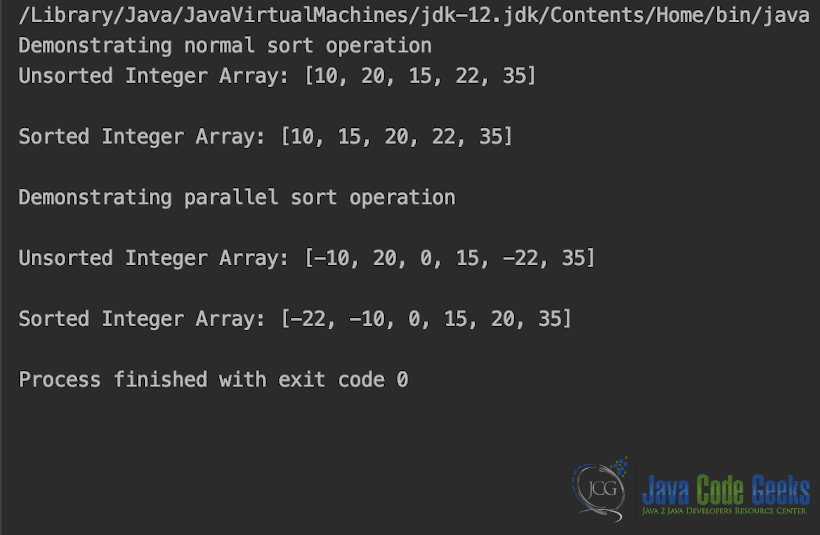
2.3 Copy
In this section we will look at the methods provided by java for Copy operations on an array. We will be using these methods to create a new array using the elements of an existing array.
2.3.1 static native void arraycopy(Object src, int srcPos, Object dest, int destPos, int length)
Copies an array from the source array, beginning at the specified position, to the specified position of the destination array.
length represents the number of array elements to be copied.
2.3.2 copyOf(T[] original, int newLength)
Returns a new array which is a copy of the array specified and padded with 0s to obtain the specified length. newLength is the number of array elements to be copied.
2.3.3 copyOfRange(T[] original, int from, int to)
Returns a new array containing the specified range from the original array, truncated or padded with nulls to obtain the required length.
we will see all versions of copy methods in action in code snippet below.
ArrayCopy.java
import java.util.Arrays;
public class ArrayCopy {
public static void main(String[] args) {
int Source[] = {5, 6, 7, 8, 9};
int Destination[] = new int[5];
System.out.println("Printing Source Array");
System.out.println(Arrays.toString(Source));
System.out.println("Printing Destination Array before copy");
System.out.println(Arrays.toString(Destination));
System.arraycopy(Source, 0, Destination, 0, 5);
System.out.println("Printing Destination Array after copy using System.arraycopy()");
System.out.println(Arrays.toString(Destination));
Arrays.fill(Destination, 0);
System.out.println("Printing Destination Array after Clearing its contents");
System.out.println(Arrays.toString(Destination));
Destination = Arrays.copyOf(Source, Source.length);
System.out.println("Printing Destination Array after copy using Arrays.copyOf()");
System.out.println(Arrays.toString(Destination));
Arrays.fill(Destination, 0);
System.out.println("Printing Destination Array after Clearing its contents");
System.out.println(Arrays.toString(Destination));
Destination = java.util.Arrays.copyOfRange(Source, 0, 5);
System.out.println("Printing Destination Array after copy using Arrays.copyOfRange()");
System.out.println(Arrays.toString(Destination));
}
}
In ArrayCopy.java
we see System.arraycopy()
, Arrays.copyOf()
and Arrays.copyOfRange()
methods in action. Output of ArrayCopy.java
can be seen in the Fig.4. below.
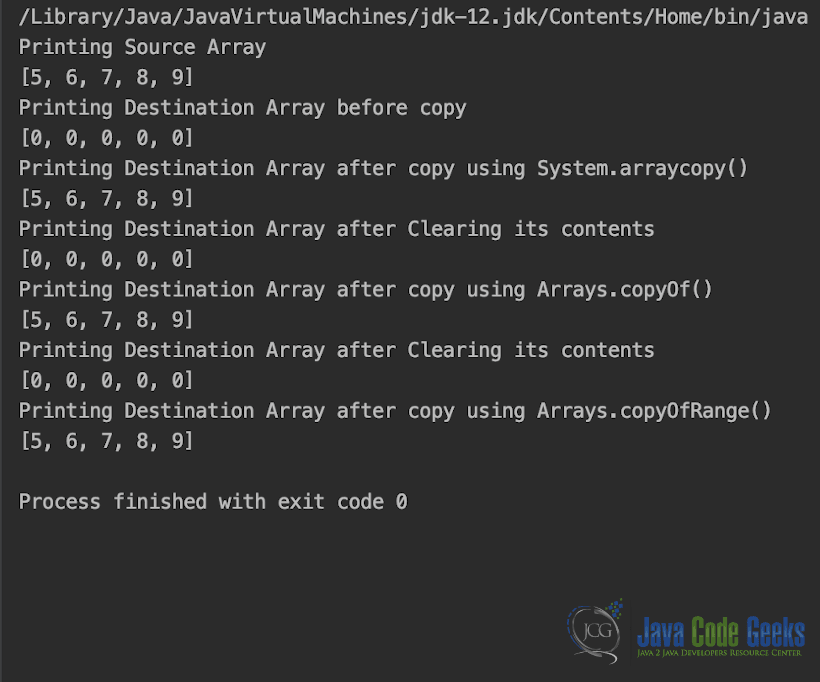
2.4 fill
In this section we will look at the methods provided by java to fill
an array. We will be using these methods to create a new array with all elements set to a custom value
2.4.1 static void fill(int[] a, int val)
Assigns the value ‘val’ to each element in the array specified.
we will see Arrays.fill()
method in action in code snippet below.
ArrayFill.java
import java.util.Arrays;
public class ArrayFill {
public static void main(String[] args) {
int[] arrayToBeFilled = new int[5];
System.out.println("arrayToBeFilled before applying Arrays.fill()");
System.out.println(Arrays.toString(arrayToBeFilled));
Arrays.fill(arrayToBeFilled, 1);
System.out.println("arrayToBeFilled after applying Arrays.fill()");
System.out.println(Arrays.toString(arrayToBeFilled));
}
}
In ArrayFill.java
we see Arrays.fill()
method in action. Output of ArrayFill.java
can be seen in the Fig.5. below.
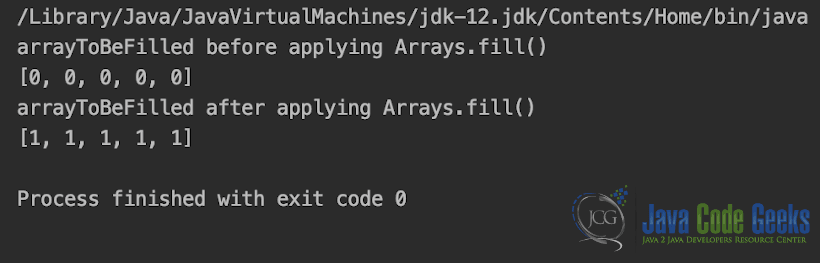
2.5 static List<Integer> asList(int[] arr)
The asList()
method of java.util.Arrays
class is returns a fixed-size list consisting of elements of the array passed as params by the specified array.
The returned list is serializable and implements RandomAccess.
2.6 static compare(int[] arr1, int[] arr2)
This method compares two arrays passed as parameters lexicographically, which basically means that the sorting will happen in alphabetical order.
2.7 static boolean equals(int[] a, int[] a2)
Returns true if the given arrays are equal. Two arrays are considered equal if both arrays contain the equal number of elements, and all corresponding pairs of elements in the two arrays are equal. This applies for the primitive data types out of the box, but when we are comparing two arrays of object off of user defined classes, then the equals()
method needs to be overriden to correctly determine whether two arrays are equal or not.
2.8 static int hashCode(int[] a)
Returns a int hashcode
for the specified array. The hashcode
is generated based off of the contents of array passed as params. For any 2 non-null integer arrays, array1 and array2, if Arrays.equals(array1, array2))
is true
then Arrays.
hashCode
(array1) == Arrays.
hashCode
(array2)
.
2.9 static String toString(<T>[] arr)
This method returns a String representation of the contents of arr
. Individual elements in string representation will be separated by comma operator.
Code example in MiscArrayMethods.java
, demonstrates toString()
, hashCode()
, equals()
, compare()
and asList()
methods is shown in the snippet below.
MiscArrayMethods.java
import java.util.Arrays;
import java.util.List;
public class MiscArrayMethods {
public static void main(String[] args) {
int[] arrayOne = new int[5];
int[] arrayTwo = new int[5];
Arrays.fill(arrayOne, 1);
Arrays.fill(arrayTwo, 1);
System.out.println("Demonstrating Arrays.equals() Method");
System.out.println(Arrays.equals(arrayOne, arrayTwo));
System.out.println("Demonstrating Arrays.compare() Method: returns 0 if both arrays are equal");
System.out.println(Arrays.compare(arrayOne, arrayTwo));
System.out.println("Demonstrating Arrays.hashCode() Method");
System.out.println("arrayOne HashCode " + Arrays.hashCode(arrayOne));
System.out.println("arrayTwo HashCode " + Arrays.hashCode(arrayTwo));
System.out.print("Are arrayOne and arrayTwo equal based on the HashCode comparison : ");
System.out.println(Arrays.hashCode(arrayOne) == Arrays.hashCode(arrayTwo));
System.out.println("Demonstrating Arrays.asList() Method");
String arrayOfStrings[] = new String[] { "A", "B", "C", "D" };
List<String> arrayListOfStrings = Arrays.asList(arrayOfStrings);
System.out.println("The list is: " + arrayListOfStrings);
System.out.println("Demonstrating Arrays.toString() Method");
System.out.println(Arrays.toString(arrayOfStrings));
}
}
Output of MiscArrayMethods.java
can be seen in the Fig.6. below.
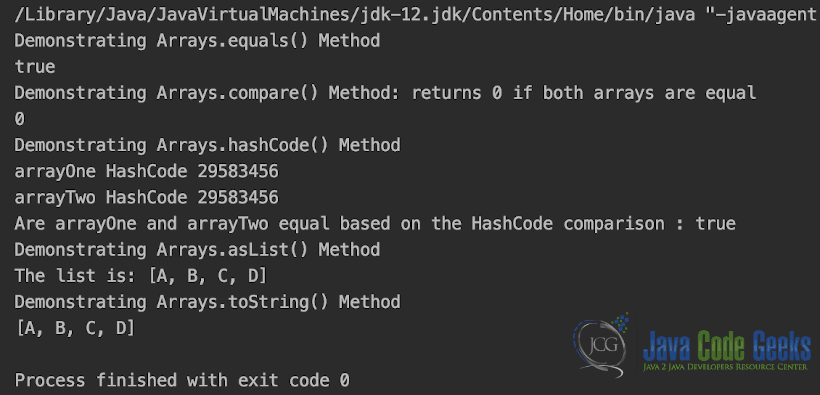
3. Updates on Array Methods in Java 8
The major addition related to arrays in java 8 was streams
. Java Streams simplifies arrays operations and at the same time increases the efficiency as well. In this article we will provide a simple example showcasing the benefits of using the Streams for aggregation operation rather than traditional iterative methods.
A code snipped show-casing the power of streams is shown in ArrayStreams.java
ArrayStreams.java
import java.util.Arrays;
public class ArrayStreams {
public static void main(String[] args) {
int[] arrayOne = {1, 2, 3, 4, 5, 6, 7, 7, 7, 8, 9};
System.out.println("Sum using the Traditional Iterative methods");
int sum = 0;
for (int i = 0; i < arrayOne.length; i++) {
sum = sum + arrayOne[i];
}
System.out.println("Sum is " + sum);
System.out.println("Sum using the Array Stream");
sum = Arrays.stream(arrayOne).sum();
System.out.println("Sum is " + sum);
}
}
output of ArrayStreams.java
is shown in Fig.7 below
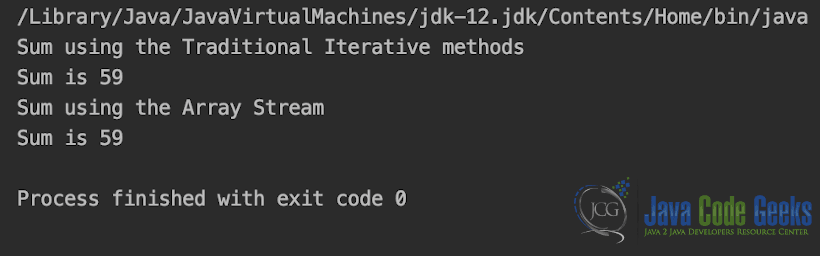
Java streams offer a lot more functions. Details of those can be found on Java Streams Official Documentations.
4. Updates on Array Methods in Java 9
A new API was introduced in Arrays
Class, mismatch()
, which two primitive arrays, and returns the index of the first differing values. If the arrays are equal then it returns -1
.
Code snippet in ArrayMisMatch.java
demonstrates the mismatch method in action.
ArrayMisMatch.java
import java.util.Arrays;
public class ArrayMisMatch {
public static void main(String[] args) {
int[] arrayOne = {2, 4, 6, 8, 10};
int[] arrayTwo = {2, 4, 6, 8, 10};
int[] arrayThree = {2, 12, 6, 8, 10};
System.out.println("arrayOne: " + Arrays.toString(arrayOne));
System.out.println("arrayTwo: " + Arrays.toString(arrayTwo));
System.out.println("arrayThree: " + Arrays.toString(arrayThree));
System.out.println("Arrays.mismatch(arrayOne, arrayTwo): " + Arrays.mismatch(arrayOne, arrayTwo));
System.out.println("Arrays.mismatch(arrayOne, arrayThree): " + Arrays.mismatch(arrayOne, arrayThree));
System.out.println("Arrays.mismatch(arrayThree, arrayOne): " + Arrays.mismatch(arrayThree, arrayOne));
}
}
output of ArrayMisMatch.java
is shown in Fig.8 below.
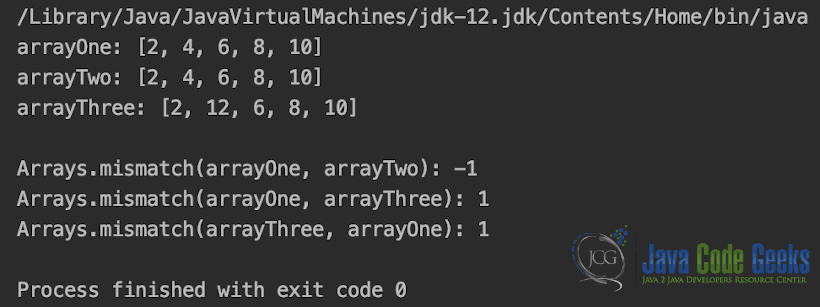
5. Summary
These are some of the most commonly used array methods. There are lot more methods exposed by java.util.Arrays
class and details for those can be find in official documentation. For further details we recommend checking out the JDK official Documentation for java.util.Arrays
class.
6. Download Source Code
You can download the full source code of this example here: Array methods Java Tutorial