Array Declaration in Java
This article is about the Array Declaration in Java. But before we initialize an array in java, we will explain one important data structure used, the Array.
1. Introduction
An array is a collection of items stored at contiguous memory locations. The idea is to store multiple items of the same type together.
We can store in an array any kind of data from primitive ones (int, double, float, boolean…) as well an object (non-primitive).
In the next sections, we going to discuss more arrays and how to implement and use them.
2. Pre-requisites
The minimum Java version for executing the article’s example is JDK 8 (find here), but we can use the most recently released Java version (JDK 15).
Also, I’m using IntelliJ 2020.2, but you can use any IDE with support for versions recommended above.
3. Array declaration
Basically, an array has two components: the type and the name.
Type declares what is the elements of the array. Also, the element type determines the data type of each element that comprises the array.
When we create an array in Java, basically we’re creating a variable that will store our data.
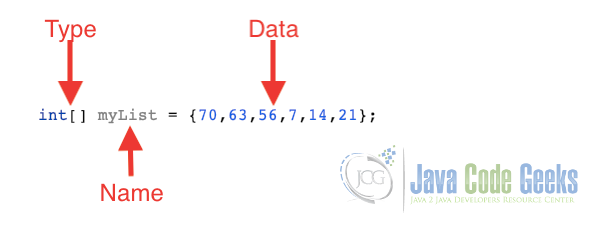
Above we can see the part a simple declaration of array in Java.
We have two kind of arrays: one-dimensional and multi-dimensional.
4. One-dimensional array
As we saw before, a one-dimensional array is declared as a variable in Java. The basic syntax to declare an array is like that:
One-dimensional array example
int[] myList; // preferred way. or int myList[]; // works but not preferred way.
To insert data in an array we can create the array with all data we want or we can create in a dynamically way, using a loop for instance.
Insert data in array
// inserting all data in declaration int[] initWithData = {7,14,21,28,35,42}; // inserting each data after declaration int[] initWithoutData = new int[6]; initWithoutData[0] = 7; initWithoutData[1] = 14; initWithoutData[2] = 21; initWithoutData[3] = 28; initWithoutData[4] = 35; initWithoutData[5] = 42; // inserting data dynamically after declaration int size = 6; int[] initDynamically = new int[size]; for(int i=0; i < initDynamically.length; i++) { initDynamically[i] = 7 * (i + 1); }
An array stores the data in index that always starts in 0. So, to go through we need to start in the position 0 of array.
Also note in the previous code that if we try to insert an incorrect position in array-like initWithoutData[6] = 48, we going to throw an exception ArrayIndexOutOfBoundsException, because the index desired is out of the array declaration (int[] initWithoutData = new int[6];
)
Note: the array index starts in 0, so the size of that array can be 6, but the last index is 5. Always discount -1 when going through an array.
5. Multi-dimensional array
We can think in a multi-dimensional array as a table with rows and columns. To clarify, think that a multi-dimensional array is an array of arrays.
Following, we going to see a 2 dimensional array declaration example:
2d array declaration
int[][] a = new int[3][4];
With the declaration above, we’ll get this kind of data stored:
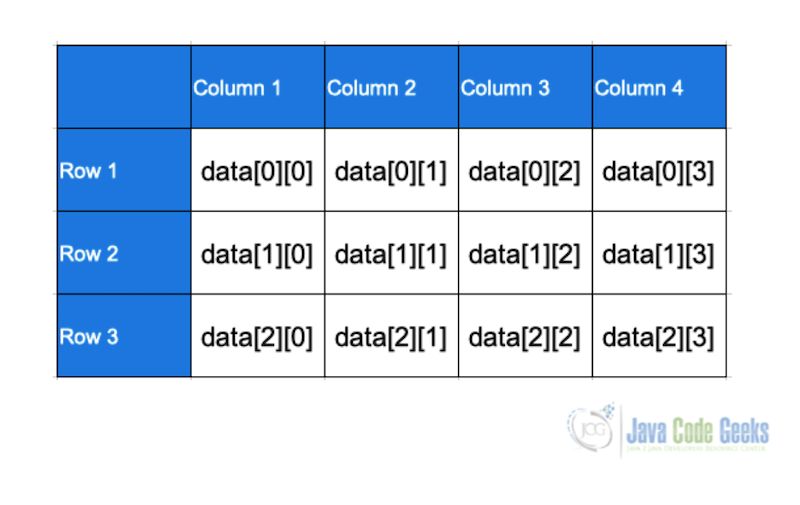
Think as the first array is the rows of the table and the second is the columns. It’s almost like a Battleship game!
Now, a code example on how we can go through that array.
2d array code example
// create a 2d array int[][] a = { {1, 2, 3}, {4, 5, 6, 9}, {7}, }; System.out.println("Print all elements of array:"); for (int i = 0; i < a.length; ++i) { System.out.println("Elements of row: " + (i + 1)); for(int j = 0; j < a[i].length; ++j) { System.out.println(a[i][j]); } }
Our data store would be like this chart on bottom:
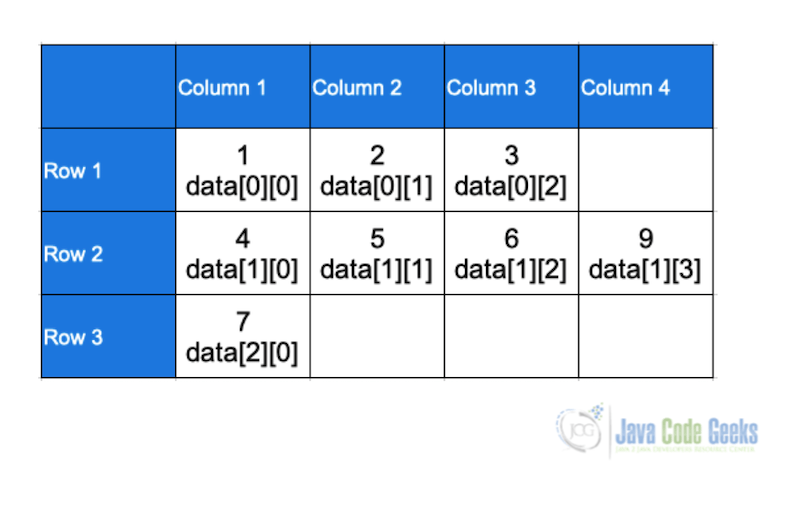
6. Summary
In conclusion, we see the data structure array in Java and how we initialize an array in java. Also, we could notice how this structure works and do some code examples with a one-dimensional array and a multi-dimensional array.
7. Download the source code
You can download the full source code of this example here: Array Declaration in Java