Apache Ant Delete Example
In this article, we will create an Apache Ant Delete Example.
1. Introduction
Ant was the first build tool that was created for building Java applications. Ant is implemented in Java, and its build scripts are written in XML. The XML build scripts were easier to understand than the “make” files at that time, so Ant quickly became popular as a build tool among Java developers. Additionally, Ant is an open-source project which made it available for free to all developers.
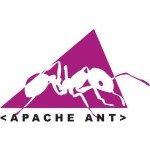
Just to recap, a “Java build tool” is a tool that can compile Java code, run the unit tests for it, package the compiled classes into a JAR file, and many, many other things. You specify what to build (and sometimes how) in a build script. This build script can then be executed again and again by the build tool. This is much easier, faster, and less error-prone than performing all these tasks manually.
2. Ant Core Concepts
The Ant core concepts are:
- Projects
- Properties
- Targets
- Tasks
- Build scripts
An Ant project contains all the information necessary to build some software projects using Ant. An Ant project is contained within an Ant build script (a file).
An Ant property is a key, value pair which you can specify. Properties typically contain information used in the build process, like directory names, file names, server names, or whatever else you may need in your project.
An Ant target typically specifies one step of the build process for the project. An Ant project contains at least one target, but more commonly an Ant project contains multiple targets. You could specify the whole build process within a single target, but typically it is easier to split the build process up into multiple targets.
Ant targets can be executed individually, so if you have multiple different build actions you need to be able to execute independently, splitting them up into multiple Ant targets is the way to go. For instance, you might want to be able to compile your code without generating JavaDoc every time, or without running the unit tests, etc. Splitting JavaDoc and unit test execution into their own targets makes this easier to achieve.
Ant tasks are build actions like copying files, compiling Java code, generating JAR files, generating JavaDoc, etc. Ant comes with a large set of built-in tasks you can use, so you don’t have to code your own tasks (but you can if you need to). An Ant target will typically contain one or more tasks. The Ant tasks perform the actual build operations of the Ant target they are listed inside.
An Ant build script contains a single Ant project, and that project may again contain properties and targets. The targets may contain tasks.
3. Technology Stack
In this example we will be using the following technology stack:
- Eclipse 4.14
- Ant 1.9.15
- JDK 1.7
4. Setting Environment Variables
To get the Ant command-line tool to work you must set a few environment variables.
First of all, you must set the JAVA_HOME environment variable to point to the directory into which you have installed your Java SDK. Note that you need a full SDK to run Ant, not just a Java Runtime Environment (JRE).
Second, you must set the ANT_HOME environment variable to point to the directory into which you unzipped the binary Ant distribution.
Third, you must add [ant-dir]/bin to the path environment variable. The [ant-dir] part should be the directory where you installed Ant (and not the text [ant-dir] literally). Since you have just set the ANT_HOME environment variable to point to the Ant install dir, you can add %ANT_HOME%/bin (on Windows) to the path variable, or ${ANT_HOME}/bin if you are using a Linux / Unix system.
5. Ant Targets
An Ant target is a sequence of tasks to be executed to perform a part (or whole) of the build process. Ant targets are defined by the user of Ant. Thus, what tasks an Ant target contains depends on what the user of Ant is trying to do in the build script. Similarly, the name of an Ant target is also decided by the creator of the Ant build script.
Some of the more commonly occurring Ant targets are:
- clean: An Ant target for cleaning up the build output directory – e.g. deleting compiled class files, deleting generated source code, or just deleting the whole build output directory completely.
- compile: An Ant target for compiling the Java source code in the project.
- jar: An Ant target for creating a JAR file from the compiled classes.
- test: An Ant target for running all unit tests for the Java code.
- Javadoc: An Ant target for creating JavaDoc comments from the Java code.
6. Ant Tasks
Ant tasks are the units of your Ant build script that actually execute the build operations for your project. Ant tasks are usually embedded inside Ant targets. Thus, when you tell Ant to run a specific target it runs all Ant tasks nested inside that target.
Some of the tasks you will see are:
- mkdir
- copy
- delete
- javac
- javadoc
- jar
In this tutorial, I will show how to build a Java project with Ant. Ant does not come with any predefined targets for building Java projects, so you will have to write your own. However, having to rewrite Ant build scripts from scratch every time you start a new Java project is tedious. Therefore this text provides a simple Ant build script for Java projects which you can use as a template for your own build scripts.
Here is the example Ant build script:
<project name="MyProject" basedir="."> <property name="version">1.2.3</property> <property name="dir.src">src/main/java</property> <property name="dir.build">build</property> <property name="dir.build.classes">${dir.build}/classes</property> <property name="dir.build.javadoc">${dir.build}/javadoc</property> <property name="file.jar">${dir.build}/MyProject-${version}.jar</property> <path id="projectClasspath"> <fileset dir="lib"> <include name="**.jar"/> </fileset> </path> <target name="clean"> <delete dir="${dir.build}"/> </target> <target name="init"> <mkdir dir="${dir.build}"/> <mkdir dir="${dir.build.classes}"/> </target> <target name="compile" depends="init"> <echo>Compiling Java source</echo> <javac classpathref="projectClasspath" srcdir="${dir.src}" destdir="${dir.build.classes}" /> </target> <target name="jar" depends="compile"> <echo>Making JAR file</echo> <jar basedir="${dir.build.classes}" file="${file.jar}" /> </target> <target name="javadoc"> <echo>Making JavaDoc from source</echo> <javadoc sourcepath="${dir.src}" destdir="${dir.build.javadoc}"></javadoc> </target> </project>
Notice how the jar target depends on the compile target, and the compile target depends on the init target. That way you are sure that all your code has been compiled when you run the jar target.
7. How to use Delete task in Apache Ant
Deletes a single file, a specified directory, and all its files and subdirectories, or a set of files specified by one or more file sets. When specifying a set of files, empty directories are not removed by default. To remove empty directories, use the includeEmptyDirs attribute.
If you use this task to delete temporary files created by editors and it doesn’t seem to work, read up on the default exclusion set in Directory-based Tasks, and see the defaultexcludesattribute below.
Parameters
Attribute | Description |
file | The file to delete, specified as either the simple filename (if the file exists in the current base directory), a relative-path filename, or a full-path filename. |
dir | The directory to delete, including all its files and subdirectories. |
verbose | Whether to show the name of each deleted file. |
includes | Comma- or space-separated list of patterns of files that must be deleted. All files are relative to the directory specified in dir |
includesfile | The name of a file. Each line of this file is taken to be an include pattern. |
excludes | Comma- or space-separated list of patterns of files that must be excluded from the deletion list. All files are relative to the directory specified in dir |
excludesfile | The name of a file. Each line of this file is taken to be an exclude pattern |
7.1 Examples
<delete file="/lib/ant.jar"/> <!--deletes the file /lib/ant.jar--> <delete dir="lib"/> <!--deletes the lib directory, including all files and subdirectories of lib--> <delete> <fileset dir="." includes="**/*.bak"/> </delete> <!--deletes all files with the extension .bak from the current directory and any subdirectories--> <delete includeEmptyDirs="true"> <fileset dir="build"/> </delete> <!--deletes all files and subdirectories of build, including build itself-->
8. Download the Source Code
In this article, we will create an Apache Ant Delete Example.
You can download the full source code of this example here: Apache Ant Delete Example