Anonymous Class Java Example
In this article, we will see examples of the anonymous class in Java language, which is a very important aspect of Programming.
1. Introduction
In java, it is possible to define a class within another class, such classes are known as nested classes. They enable you to logically group classes that are only used in one place, thus this increases the use of encapsulation, and create more readable and maintainable code.
Anonymous Inner Class is an inner class without a name and for which only a single object is created. It can be useful when making an instance of an object with certain “extras” such as overloading methods of a class or interface, without having to subclass a class.
2. Syntax of Anonymous Classes
These classes are inner classes with no name. Since they have no name, we can’t use them to create instances of anonymous classes. As a result, we have to declare and instantiate anonymous classes in a single expression at the point of use.
We may either extend an existing class or implement an interface.
2.1. Extend a Class
In the parentheses, we specify the parameters that are required by the constructor of the class that we are extending.
new Book("Design Patterns") { @Override public String description() { return "Famous GoF book."; } }
2.2. Implement an Interface
Java’s interfaces have no constructors, so the parentheses always remain empty. This is the only way we should do it to implement the interface’s methods.
new Runnable() { @Override public void run() { ... } }
3. Anonymous Class Types
Based on declaration and behavior, there are 3 types of these Inner classes:
3.1. Anonymous Inner class that extends a class
We can have an anonymous inner class that extends a class.
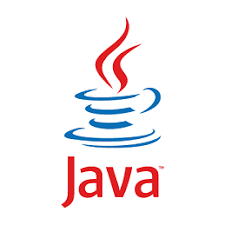
For example, we know that we can create a thread by extending a Thread class. Suppose we need an immediate thread but we don’t want to create a class that extends Thread class all the time. With the help of this type of Anonymous Inner class we can define a ready thread as follows:
//Java program to illustrate creating an immediate thread //Using Anonymous Inner class that extends a Class public class MyThread { public static void main(String[] args) { //Here we are using Anonymous Inner class //that extends a class i.e. Here a Thread class Thread t = new Thread() { public void run() { System.out.println("Child Thread"); } }; t.start(); System.out.println("Main Thread"); } }
3.2. Anonymous Inner class that implements an interface
We can also have an anonymous inner class that implements an interface.
For example, we also know that by implementing Runnable interface we can create a Thread. Here we use anonymous Inner class that implements an interface.
//Java program to illustrate defining a thread //Using Anonymous Inner class that implements an interface public class MyThread { public static void main(String[] args) { //Here we are using Anonymous Inner class //that implements a interface i.e. Here Runnable interface Runnable r = new Runnable() { public void run() { System.out.println("Child Thread"); } }; Thread t = new Thread(r); t.start(); System.out.println("Main Thread"); } }
3.3. Anonymous Inner class that defines inside method/constructor argument
Anonymous inner classes in method/constructor arguments are often used in graphical user interface (GUI) applications.
//Java program to illustrate defining a thread //Using Anonymous Inner class that define inside argument public class MyThread { public static void main(String[] args) { //Here we are using Anonymous Inner class //that define inside argument, here constructor argument Thread t = new Thread(new Runnable() { public void run() { System.out.println("Child Thread"); } }); t.start(); System.out.println("Main Thread"); } }
4. Differences between anonymous and regular inner clas
- A normal class can implement any number of interfaces but an anonymous inner class can implement only one interface at a time.
- A regular class can extend a class and implement any number of interface simultaneously. But this Inner class can extend a class or can implement an interface but not both at a time.
- For regular/normal class, we can write any number of constructors but we can’t write any constructor for this Inner class because the anonymous class does not have any name and while defining constructor class name and constructor name must be same.
5. Anonymous Class Use Cases
There might be a big variety of applications of these classes. Let’s explore some possible use cases.
5.1. Class Hierarchy and Encapsulation
We should use inner classes in general use cases and these ones in very specific ones to achieve a cleaner hierarchy of classes in our application. When using inner classes, we may achieve a finer encapsulation of the enclosing class’s data. If we define the inner class functionality in a top-level class, then the enclosing class should have public or package visibility of some of its members. Naturally, there are situations when it is not very appreciated or even accepted.
5.2. Cleaner Project Structure
We usually use these classes when we have to modify on the fly the implementation of methods of some classes. In this case, we can avoid adding new java files to the project to define top-level classes. This is especially true if that top-level class would be used just one time.
5.3. UI Event Listeners
In applications with a graphical interface, the most common use case of these classes is to create various event listeners.
6. Summary
In this article, we’ve considered various aspects of Java anonymous classes.
This classes also have the same restrictions as local classes to their members:
- We cannot declare static initializers or member interfaces in an anonymous class.
- This class can have static members provided that they are constant variables
Like local classes, these classes can capture variables; they have the same access to local variables of the enclosing scope:
- This class has access to the members of its enclosing class.
- This class cannot access local variables in its enclosing scope that are not declared as final or effectively final.
- Like a nested class, a declaration of a type (such as a variable) in an anonymous class shadow any other declarations in the enclosing scope that have the same name.
7. Download the Source Code
You can download the full source code of this example here: Anonymous Class Java Example
Good