Access Modifiers in Java Example
In this post, we will talk about the types of Access Modifiers in Java, which are private, default, protected, and public.
Generally, there are two types of modifiers in Java: access modifiers and non-access modifiers.
The access modifiers specify accessibility (scope) of a class, constructor, variable, method, or data member.
1. Access Modifiers in Java – Types
We have 4 types of java access modifiers:
- private
- default
- protected
- public
There are many non-access modifiers such as:
- static
- abstract
- synchronized
- native
- volatile
- transient
In this article we will learn access modifiers.
1.1 Private access modifier
The private access modifier is specified using the keyword private.
- The methods or data members declared as private are accessible only within the class in which they are declared.
- Any other class of the same package will not be able to access these members.
- Top level Classes or interface can not be declared as private because
- private in Javameans “only visible within the enclosing class”.
- protected in Java means “only visible within the enclosing class and any subclasses”
Hence these modifiers in terms of application to classes, they apply only to nested classes and not on top-level classes
1.1.1 Example of private access modifier
In this example, we have created two classes A and B within same package package. A contains private data member and private method. We try to access the private method of A from class B and see the result. There will be compile time error.
Below you can find two classes that are illustrating an error while using class with private modifier:
class A_privateModifier
package projectPackage; public class A { private int data=40; private void print(){ System.out.println("Data is:" + data); } }
class B_privateModifier
package projectPackage; public class B { public static void main(String args[]){ A instance=new A(); System.out.println(instance.data);//Compile Time Error //trying to access private method of class A instance.print();//Compile Time Error } }
As you can see in the picture below:
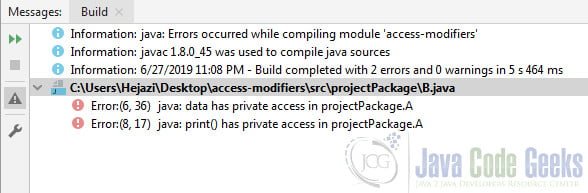
If you make any class constructor private, you cannot create the instance of that class from outside the class:
class A_private constructor:
public class A { private int data=40; private A(){}; //private constructor private void print(){ System.out.println("Data is:" + data); } }
class B_private constructor:
public class B { public static void main(String args[]){ A instance=new A(); //Compile Time Error } }
As you can see, we get the following error:
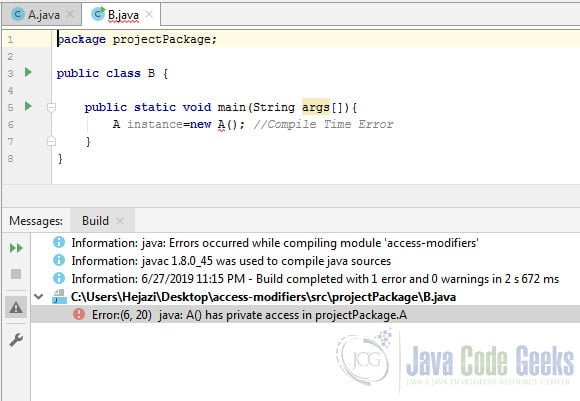
Note: In java, a class cannot be private or protected except nested class.
1.2 Default access modifier
When no access modifier is specified for a class , method or data member it is treated as default. The scope of this modifier is limited to the package only so it is accessible only within the same package. This means that if we have a class with the default access modifier in a package, only those classes that are in this package can access this class.
1.2.1 Example of default access modifier
In this example, we have created two packages packA and packB. We are accessing the A class from outside its package, since A class is not public, so it cannot be accessed from outside the package.
class A in packA_default modifier:
package packA; //Class A is having Default access modifier class A { void print(){ System.out.println("This is A"); } }
class B in packB_default modifier:
package packB; import packA.A; //Class B is having Default access modifier public class B { public static void main(String args[]){ //accessing class A from package packA A instance = new A();//Compile Time Error instance.print();//Compile Time Error } }
We will get these errors:
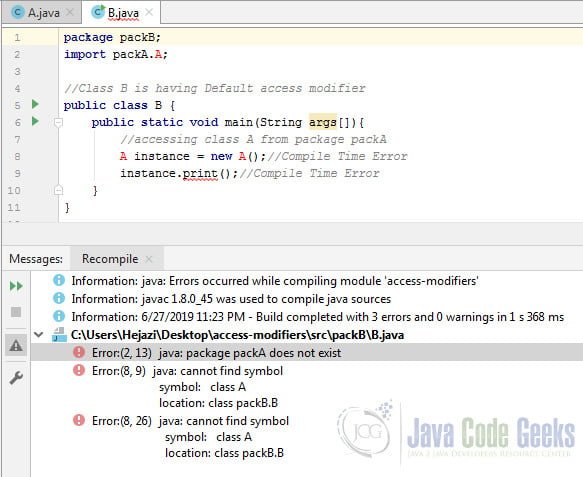
1.3 Protected access modifier
The protected access modifier is accessible within same package and outside the package but through inheritance only.
The protected access modifier can be applied on the data member, method and constructor. It can’t be applied on the class.
1.3.1 Example of protected access modifier
In this example, we have created the two packages packA and packB. The A class of packA package is public, so can be accessed from outside the package. But print method of this package is declared as protected, so it can be accessed from outside the class only through inheritance.
class A in packA_
protected modifier
package packA; public class A { protected void print(){ System.out.println("This is A"); } }
class B in packB_
protected modifier
package packB; import packA.A; class B extends A { public static void main(String args[]){ B instance = new B(); instance.print(); } }
Output: This is A
1.4 Public access modifier
The public access modifier is accessible everywhere. There is no restriction on the scope of a public data members.
1.4.1 Example of public access modifier
In this example we have public class A in Package A and a default class B in Package B in which we can access to the method of class A without even extending class A.
class A in packA_
public modifier
package packA; public class A { public void print(){ System.out.println("This is A"); } }
class B in packB_
public modifier
package packB; import packA.A; class B { public static void main(String args[]){ A instance = new A(); instance.print(); } }
Output: This is A
All we have discused so far is summorized in the table below:
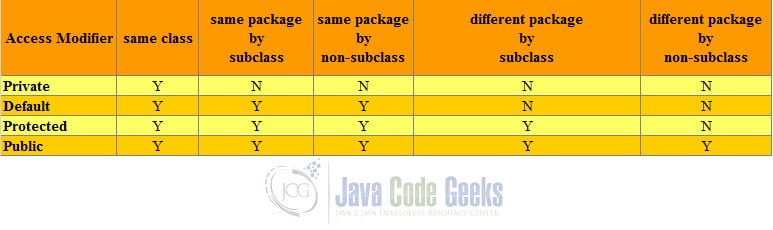
2. Java access modifiers with method overriding
If you are overriding any method, overridden method (i.e. declared in subclass) must not be more restrictive.
class A
class A{ protected void print(){System.out.println("This is A");} }
class B_more restrictive overriden method
public class B extends A{ void print(){System.out.println("This is B");}//Compile Time Error public static void main(String args[]){ B instance=new B(); instance.print(); } }
The default modifier is more restrictive than protected. So we get compile time error.
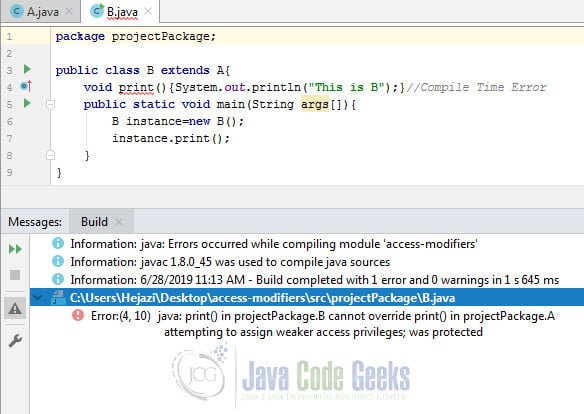
3. Download the Complete Source Code
You can download the full source code of this example here: Access Modifiers in Java Example
Last updated on Aug 26, 2019
Default is also a non-access modifier, used in interfaces since Java 8.