Java this Keyword Example
In this article, we will try to understand the Java this keyword with the help of examples.
this, self, and Me are keywords used in some computer programming languages to refer to the object, class, or other entity of which the currently running code is a part. The entity referred to by these keywords thus depends on the execution context (such as which object is having its method called). Different programming languages use these keywords in slightly different ways. In languages where a keyword like “this” is mandatory, the keyword is the only way to access data and methods stored in the current object. Where optional, they can disambiguate variables and functions with the same name.
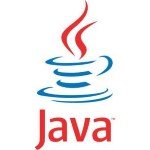
The this keyword refers to the current object in a method or constructor. Any member of the current object can be referenced from within a non static method or a constructor by using this.
All examples in this article were created using Eclipse Photon IDE and Java 8.
1. Usages of Java this Keyword
The following are the usages of this keyword.
1.1 Usage of this to access the current object variable
The following example shows how the shadowing (two variables with the same name within scopes that overlap) of the variable description is avoided by using this.
ThisKeywordVariableExample.java
package com.javacodegeeks.example.keywords; public class ThisKeywordVariableExample { private String description; public void printDescription(String description) { this.description= "bar"; System.out.println("The value of method local variable = "+ description); System.out.println("The value of instance variable = "+this.description); } public static void main(String[] args) { ThisKeywordVariableExample obj = new ThisKeywordVariableExample(); obj.printDescription("foo"); } }
Output
The value of method local variable = foo The value of instance variable = bar
1.2 Usage of this to invoke a constructor
When there are overloaded constructors in a class, from within a constructor this has to be used in order to call another constructor as it can not be called explicitly. Also, note that this keyword has to be the first statement in a constructor. A constructor can have either this or super but not both.
ThisKeywordConstructorExample.java
package com.javacodegeeks.example.keywords; public class ThisKeywordConstructorExample { String a; String b; ThisKeywordConstructorExample() { this("foo","bar"); System.out.println("Inside no argument constructor"); } ThisKeywordConstructorExample(String a, String b) { this.a=a; this.b=b; System.out.println("Inside parameterized constructor "+a+", "+b); } public static void main(String[] args) { ThisKeywordConstructorExample obj = new ThisKeywordConstructorExample(); } }
Output
Inside parameterized constructor foo, bar Inside no argument constructor
1.3 Usage of this to make a method call.
The this keyword can be used to invoke a method from another method in the same class.
ThisToInvokeMethod.java
package com.javacodegeeks.example.keywords; public class ThisToInvokeMethod { public void method1() { System.out.println("Method1 invoked"); this.method2(); } public void method2() { System.out.println("Method2 invoked"); } public static void main(String[] args) { ThisToInvokeMethod thisToInvokeMethod = new ThisToInvokeMethod(); thisToInvokeMethod.method1(); } }
Output
Method1 invoked Method2 invoked
1.4 Usage of this as a parameter to a method
The this keyword can be used to pass the instance of the current class to a method as a method argument.
ThisAsMethodParamExample.java
package com.javacodegeeks.example.keywords; public class ThisAsMethodParamExample { public void printClassInstance(ThisAsMethodParamExample obj) { System.out.println("Runtime class of the object = "+obj.getClass()); } public void passObjectInstance() { printClassInstance(this); } public static void main(String[] args) { ThisAsMethodParamExample thisAsMethodParamExample = new ThisAsMethodParamExample(); thisAsMethodParamExample.passObjectInstance(); } }
Output
Runtime class of the object = class com.javacodegeeks.example.keywords.ThisAsMethodParamExample
1.5 Usage of this as an argument in Constructor call
The this keyword can be used to pass the instance of the current class to a constructor as an argument.
ThisAsConstructorArgExample.java
package com.javacodegeeks.example.keywords; public class ThisAsConstructorArgExample { SupportingClass obj; // Parameterized constructor with object of SupportingClass // as a parameter ThisAsConstructorArgExample(SupportingClass obj) { this.obj = obj; // calling display method of SupportingClass obj.display(); } } class SupportingClass { String str = "foo"; // Default Contructor that creates an object of ThisAsConstructorArgExample // passing this as an argument in the constructor SupportingClass() { ThisAsConstructorArgExample obj = new ThisAsConstructorArgExample(this); } void display() { System.out.println("Value of str in Supporting Class : " + str); } public static void main(String[] args) { SupportingClass obj = new SupportingClass(); } }
Output
Value of str in Supporting Class : foo
1.6 Usage of this with Cloneable
The following example demonstrates the usage of this in a practical application (this in the context of Cloneable).
ThisKeywordCloneExample.java
package com.javacodegeeks.example.keywords; public class ThisKeywordCloneExample implements Cloneable{ private Long id; private String name; private boolean isClone; @Override protected ThisKeywordCloneExample clone() throws CloneNotSupportedException { ThisKeywordCloneExample clonedObject = new ThisKeywordCloneExample(); clonedObject.setId(this.getId()); clonedObject.setName(this.getName()); clonedObject.setClone(true); return clonedObject; } public Long getId() { return id; } public void setId(Long id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public boolean isClone() { return isClone; } public void setClone(boolean isClone) { this.isClone = isClone; } @Override public String toString() { return "[id=" + id + ", name=" + name + ", isClone=" + isClone + "]"; } public static void main(String[] args) { ThisKeywordCloneExample object1 = new ThisKeywordCloneExample(); object1.setId(123L); object1.setName("foo"); System.out.println("Original object = "+object1.toString()); try { ThisKeywordCloneExample clonedObject = object1.clone(); System.out.println("Cloned object = "+clonedObject.toString()); } catch (CloneNotSupportedException e) { e.printStackTrace(); } } }
Output
Original object = [id=123, name=foo, isClone=false] Cloned object = [id=123, name=foo, isClone=true]
1.7 Keyword this in a static context
The keyword this can not be used in a static context. The following code will not compile.
ThisStaticExample.java
package com.javacodegeeks.example.keywords; public class ThisStaticExample { private String name; public static void display() { // Compile time error which states - cannot use this in a static context System.out.println(this.name); } }
1.8 Keyword this can not be assigned value
The keyword this can not be assigned a value. The following code will not compile.
ThisAssignValueExample.java
package com.javacodegeeks.example.keywords; public class ThisAssignValueExample { public void getProductType(String productCode) { if("Copper".equalsIgnoreCase(productCode)) { //compile time error which states - LHS of an assignment must be a variable this = new CopperProduct(); } } }
2. Download the Source Code
That was an example about this Keyword.
You can download the full source code of this example here: Java this Keyword Example