2D Array Java Example
In this post, we feature a comprehensive 2D Array Java Example. Java support one dimensional, two dimensional and generally multidimensional arrays.
1. Introduction
An array is a container object that holds a fixed number of values of a single type. The length of an array is established when the array is created.
Java gives us the opportunity of using arrays with many dimensions. The syntax to declare a multidimensional array is as:
{Datatype}[1D][2D][..][ND]
You can watch the following video and learn how to use arrays in Java:
The most common multidimensional arrays that are used in java apps are one (1D), two (2D) and three (3D) dimensional. 2D
array represents a tabular data in row and column style. The first bracket is for the rows and the second bracket is for the column. E.g. int[3][2]
declares a 2D integer array with 3 rows and 2 columns.
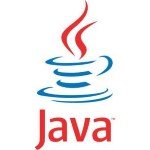
[rowIdx][colIdx] | column 0 | column 1 |
row 0 | item[0][0] | item[0][1] |
row 1 | item[1][0] | item[1][1] |
row 2 | item[2][0] | item[2][1] |
In this example we are going to demonstrate:
- Declare a 2-D array
- Declare and create a 2-D array
- Declare, create, and initialize a 2-D array
- Declare, create, and assign the value to a 2-D array’s element
- Print a 2-D array
- Show ArrayIndexOutBoundException
2. Technologies Used
The example code in this article was built and run using:
- Java 11
- Maven 3.3.9
- Junit 4.12
- Eclipse Oxygen
3. Maven Project
In this step, I will create a Maven project which includes 2D array examples.
3.1 Dependencies
I will include Junit
in the pom.xml
.
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>jcg.zheng.demo</groupId> <artifactId>java-2dArray-demo</artifactId> <version>0.0.1-SNAPSHOT</version> <build> <sourceDirectory>src</sourceDirectory> <plugins> <plugin> <artifactId>maven-compiler-plugin</artifactId> <version>3.8.0</version> <configuration> <release>11</release> </configuration> </plugin> </plugins> </build> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.12</version> </dependency> </dependencies> </project>
3.2 Card
In this example, I will create a Card
class to be used to create a 2D array with Card
type.
Card.java
package jcg.zheng.demo.data; public class Card { public Card(String suit, String rank) { super(); this.suit = suit; this.rank = rank; } private String suit; private String rank; public String getSuit() { return suit; } public void setSuit(String suit) { this.suit = suit; } public String getRank() { return rank; } public void setRank(String rank) { this.rank = rank; } @Override public String toString() { return "[" + rank + " of " + suit + "]"; } }
4. JUnit Test
4.1. 2D Integer Array
In this step, I will create a java class named TwoDIntArrayTest
with the following methods:
declare_array
– defines a 2-D array with an integer type.declare_and_create_array
– defines a 2-D integer array with 4 rows. The columns arenull
objects.declare_and_create_array_with_column
– defines a 2-D integer array with 4 rows and 2 columns. The columns are 1-D array with default value as 0.declare_create_and_initialize_array
– defines a 2-D integer array with initial values.declare_create_assign_array
– defines a 2-D integer array and allocates with a row size and assigns the columns for each row.print2DIntArray
– prints out a 2-D integer array’s elements.- array_has_boundary – shows the array has a fixed size, it will throw an exception when accessing an array out of its boundary.
TwoDIntArrayTest.java
package jcg.zheng.demo.array; import static org.junit.Assert.assertEquals; import static org.junit.Assert.assertNotNull; import static org.junit.Assert.assertNull; import org.junit.Test; public class TwoDIntArrayTest { private static final int COLUMN_COUNT = 4; private static final int ROW_COUNT = 2; @Test public void declare_array() { int[][] anArray; System.out.println("done"); } @Test public void declare_and_create_array() { int[][] anArray = new int[ROW_COUNT][]; for (int i = 0; i < ROW_COUNT; i++) { assertNull(anArray[i]); } } @Test public void declare_and_create_array_with_column() { int[][] anArray = new int[ROW_COUNT][COLUMN_COUNT]; for (int i = 0; i < ROW_COUNT; i++) { assertNotNull(anArray[i]); for (int j = 0; j < COLUMN_COUNT; j++) { assertEquals(0, anArray[i][j]); } } } @Test public void declare_create_and_initialize_array() { int[][] tableInt = new int[][] { { 1, 2, 3 }, { 6, 7, 8 }, { 5, 6, 7 } }; print2DIntArray(tableInt); } @Test public void declare_create_assign_array() { int[][] tableInt = new int[ROW_COUNT][]; for (int i = 0; i < ROW_COUNT; i++) { int[] row = new int[COLUMN_COUNT]; tableInt[i] = row; for (int j = 0; j < COLUMN_COUNT; j++) { assertEquals(0, tableInt[i][j]); } } } private void print2DIntArray(int[][] tableInt) { for (int i = 0; i < tableInt.length; i++) { for (int j = 0; j < tableInt[i].length; j++) { System.out.printf("tableInt[%d][%d] = %d\n", i, j, tableInt[i][j]); } System.out.println(); } } @Test(expected = ArrayIndexOutOfBoundsException.class) public void array_has_boundary() { int[] test = new int[ROW_COUNT]; test[test.length] = 4; } }
As you can see, this manner is very similar to the declaration of one-dimensional array. Execute mvn test -Dtest=TwoDIntArrayTest
and capture the output here.
Output
------------------------------------------------------- T E S T S ------------------------------------------------------- Running jcg.zheng.demo.array.TwoDIntArrayTest done tableInt[0][0] = 1 tableInt[0][1] = 2 tableInt[0][2] = 3 tableInt[1][0] = 6 tableInt[1][1] = 7 tableInt[1][2] = 8 tableInt[2][0] = 5 tableInt[2][1] = 6 tableInt[2][2] = 7 Tests run: 6, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.172 sec Results : Tests run: 6, Failures: 0, Errors: 0, Skipped: 0 [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 10.678 s [INFO] Finished at: 2019-11-01T11:30:11-05:00 [INFO] ------------------------------------------------------------------------ C:\MaryZheng\Workspaces\jdk12\java-2dArray-demo>
4.2 2D Object Array
Instead of declaring only primitive types in an array, we can use objects too. In this example, I will create TwoDObjectarrayTest
class to cover the definition of Card
2D array. Every cell of this 2-dimensional array represents an instance of Card
class.
declare_and_create_2D_array_default_null
– defines a 2DCard
array with 4 rows and 2 columns and verifies the default elements arenull
objects.declare_create_initialize_string_2d_array
– defines, creates, and initializes a 2DString
array.print2DArray
– prints a 2D array elements with twofor
loopsdeal_poker_card_game
– illustrates a 2DCard
array with a poker game. It draws 5 cards for 4 players and prints out players’ hand.
TwoDObjectArrayTest.java
package jcg.zheng.demo.array; import static org.junit.Assert.assertEquals; import org.junit.Test; import jcg.zheng.demo.data.Card; public class TwoDObjectArrayTest { private static final int COLUMN_COUNT = 4; private static final int ROW_COUNT = 2; @Test public void declare_and_create_2D_array_default_null() { Card[][] array_2d = new Card[ROW_COUNT][COLUMN_COUNT]; for (int i = 0; i < ROW_COUNT; i++) { for (int j = 0; j < COLUMN_COUNT; j++) { assertEquals(null, array_2d[i][j]); } } } @Test public void declare_create_initialize_String_2d_array() { String[][] array_2d = new String[][] { { "apple", "orange" }, { "kiwi", "strawberry" }, { "cranberry", "grape" } }; print2DArray(array_2d); } private void print2DArray(Object[][] array_2d) { for (int i = 0; i < array_2d.length; i++) { for (int j = 0; j < array_2d[i].length; j++) { System.out.printf("[%d][%d]=%s ", i, j, array_2d[i][j]); } System.out.println(); } } @Test public void deal_poker_card_game() { // 4 players play one game of poker int PLAYER_COUNT = 4; int HAND_CARD_COUNT = 5; Card[][] cards = new Card[HAND_CARD_COUNT][PLAYER_COUNT]; // draw first card for 4 players cards[0][0] = new Card("Spade", "1"); cards[0][1] = new Card("Heart", "J"); cards[0][2] = new Card("Diamond", "3"); cards[0][3] = new Card("Club", "K"); // draw second card for 4 players cards[1][0] = new Card("Heart", "1"); cards[1][1] = new Card("Spade", "10"); cards[1][2] = new Card("Club", "5"); cards[1][3] = new Card("Diamond", "8"); // draw third card for players cards[2][0] = new Card("Spade", "11"); cards[2][1] = new Card("Heart", "A"); cards[2][2] = new Card("Diamond", "8"); cards[2][3] = new Card("Club", "K"); // draw fourth card for players cards[3][0] = new Card("Heart", "9"); cards[3][1] = new Card("Spade", "2"); cards[3][2] = new Card("Club", "Q"); cards[3][3] = new Card("Diamond", "7"); // draw fifth card for players cards[4][0] = new Card("Heart", "5"); cards[4][1] = new Card("Spade", "K"); cards[4][2] = new Card("Club", "10"); cards[4][3] = new Card("Diamond", "4"); print2DArray(cards); // print out each player's hand for (int playerIdx = 0; playerIdx < PLAYER_COUNT; playerIdx++) { System.out.printf("\nPlayer " + (playerIdx + 1) + " hand :"); for (int cardIndex = 0; cardIndex < HAND_CARD_COUNT; cardIndex++) { System.out.print(cards[cardIndex][playerIdx] + " "); } } } }
For a better understanding, you can see the output of the execution of the above code.
Output
------------------------------------------------------- T E S T S ------------------------------------------------------- Running jcg.zheng.demo.array.TwoDObjectArrayTest [0][0]=[1 of Spade] [0][1]=[J of Heart] [0][2]=[3 of Diamond] [0][3]=[K of Club] [1][0]=[1 of Heart] [1][1]=[10 of Spade] [1][2]=[5 of Club] [1][3]=[8 of Diamond] [2][0]=[11 of Spade] [2][1]=[A of Heart] [2][2]=[8 of Diamond] [2][3]=[K of Club] [3][0]=[9 of Heart] [3][1]=[2 of Spade] [3][2]=[Q of Club] [3][3]=[7 of Diamond] [4][0]=[5 of Heart] [4][1]=[K of Spade] [4][2]=[10 of Club] [4][3]=[4 of Diamond] Player 1 hand :[1 of Spade] [1 of Heart] [11 of Spade] [9 of Heart] [5 of Heart] Player 2 hand :[J of Heart] [10 of Spade] [A of Heart] [2 of Spade] [K of Spade] Player 3 hand :[3 of Diamond] [5 of Club] [8 of Diamond] [Q of Club] [10 of Club] Player 4 hand :[K of Club] [8 of Diamond] [K of Club] [7 of Diamond] [4 of Diamond] [0][0]=apple [0][1]=orange [1][0]=kiwi [1][1]=strawberry [2][0]=cranberry [2][1]=grape Tests run: 3, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.401 sec Results : Tests run: 3, Failures: 0, Errors: 0, Skipped: 0 [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 10.294 s [INFO] Finished at: 2019-11-01T11:33:18-05:00 [INFO] ------------------------------------------------------------------------ C:\MaryZheng\Workspaces\jdk12\java-2dArray-demo>
5. Summary
In this example, I showed how to declare, create, and initialize a 2D array with both integer and Card
. Please remember that Array
has fixed-size elements and will throw an ArrayIndexOutBoundException
if accessing an index which is outside of the boundary.
Please click here for how to add and remove an element and click here for how to copy an array.
6. Download the Source File
This example consists of a Maven project which includes a tutorial about 2D array for declaration, creation, initialization, assigning, accessing, and retrieving.
Download the source code of this example: 2D Array Java Example
Last updated on Nov. 5th, 2019